The platform module provides an API to get information about the underlying system/platform where our code runs. Information such as OS name, Python Version, Architecture, Hardware information, etc. is exposed via platform module functions. This module does not require installation since it is part of the default libraries that comes with python installation.
First, let’s Import the “platform” module.
# python3 >>> import platform >>> print("Imported Platform module version: ", platform.__version__)
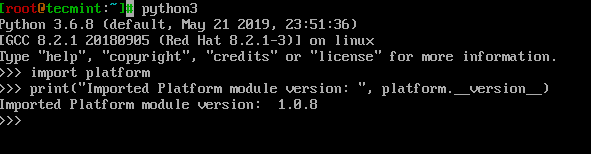
Let’s grab some information about python first, like what is the version, build information, etc..
- python_version() – Returns the python version.
- python_version_tuple() – Returns python version in tuple.
- python_build() – Returns build number and date in the form of a tuple.
- python_compiler() – Compiler used to compile python.
- python_implementation() – Returns python implementation like “PyPy”,”CPython”, etc..
>>> print("Python version: ",platform.python_version()) >>> print("Python version in tuple: ",platform.python_version_tuple()) >>> print("Build info: ",platform.python_build()) >>> print("Compiler info: ",platform.python_compiler()) >>> print("Implementation: ",platform.python_implementation())
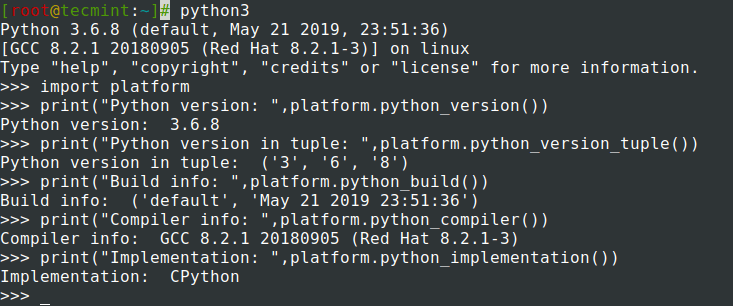
Now let’s grab some system-related information, like OS flavor, release version, processor, etc..
- system() – Returns system/OS name like “Linux”, “Windows”, “Java”.
- version() – Returns system version information.
- release() – Returns the system release version.
- machine() – Returns machine type.
- processor() – Returns system processor name.
- node() – Returns the system network name.
- platform() – Returns as much as useful information about the system.
>>> print("Running OS Flavour: ",platform.system()) >>> print("OS Version: ",platform.version()) >>> print("OS Release: ",platform.release()) >>> print("Machine Type: ",platform.machine()) >>> print("Processor: ",platform.processor()) >>> print("Network Name: ",platform.node()) >>> print("Linux Kernel Version: ",platform.platform())
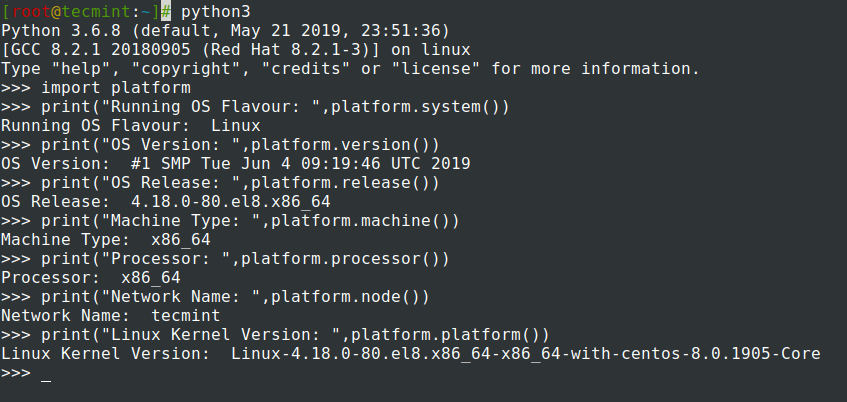
Instead of accessing all the system-related information through separate functions, we can use the uname()
function which returns a named tuple with all the information like System Name, release, Version, machine, processor, node. We can use index values to access specific information.
>>> print("Uname function: ",platform.uname()) >>> print("\nSystem Information: ",platform.uname()[0]) >>> print("\nNetwork Name: ",platform.uname()[1]) >>> print("\nOS Release: ",platform.uname()[2]) >>> print("\nOS Version: ",platform.uname()[3]) >>> print("\nMachine Type: ",platform.uname()[4]) >>> print("\nMachine Processor: ",platform.uname()[5])
Think of a use case where you want to run your program only in a certain version of python or only in a specific OS flavor, In that case, the platform module is very handy.
Below is a sample pseudocode to check the python version and OS flavor.
import platform import sys if platform.python_version_tuple()[0] == 3: < Block of code > else: sys.exit() if platform.uname()[0].lower() == "linux": < Block of Code > else: sys.exit()
Python Keyword Module
Every programming language comes with built-in keywords that servers different functionality. For eg: True, False, if, for, etc.. Similarly, python has built-in keywords that cannot be used as identifiers to variable, functions, or class.
The keyword module provides 2 functionality.
- kwlist – Prints out the list of built-in keywords.
- iskeyword(s) – Returns true if s is a python defined keyword.
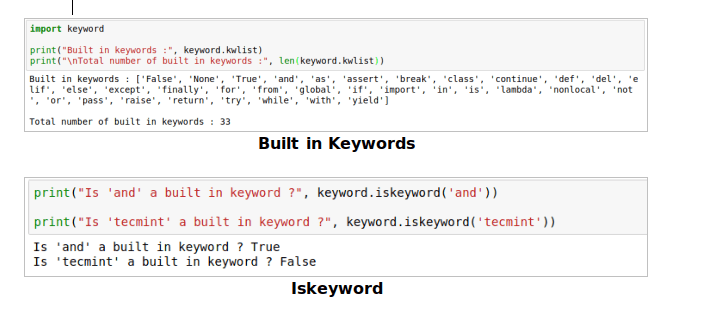
Now that we have come to the end of the article, so far we have discussed 2 python modules (Platform and Keyword). The platform module is very useful when we want to grab some information about the system we are working with. On the other hand, the keyword module provides a list of built-in keywords and functions to check if a given identifier is a keyword or not.