Creating applications on Linux can be done using different ways, but there are a limited ways of doing, so using the simplest and the most functional programming languages and libraries, that’s why we’re going to have a quick look about creating applications under the Linux desktop using the GTK+ library with Python programming language which is called “PyGObject”.
PyGObject uses the GObject Introspection to create binding for programming languages like Python, PyGObject is the next generation from PyGTK, you can say that PyGObject = Python + GTK3.
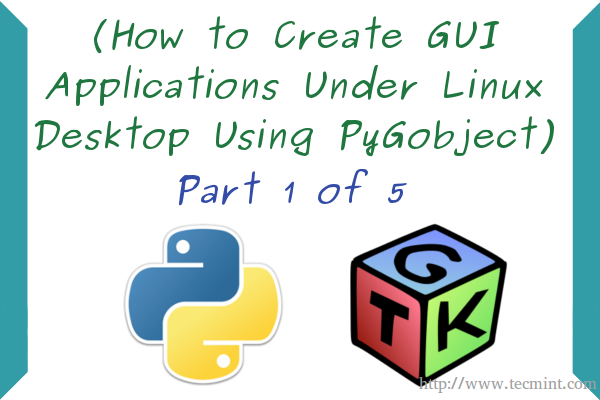
Today, we’re going to start a series about creating GUI (Graphical User Interface) applications under the Linux desktop using GTK+ library and PyGobject language, the series will cover the following topics:
About Python
First of all, you must have some basic knowledge in Python; Python is a very modern and easy to use programming language. It’s one of the most famous programming languages in the world, using Python, you will be able to create many great applications & tools. You may take some free courses like those at codeacademy.com or you may read some books about Python at:
About GTK+
GTK+ is an open-source cross-platform toolkit to create graphical user interfaces for desktop applications, it was first started in 1998 as a GUI toolkit for the GIMP, later, it was used in many other applications and soon became one of the most famous libraries to create GUIs. GTK+ is released under the LGPL license.
Creating GUI Applications Under Linux
There are 2 ways for creating the applications using GTK+ & Python:
- Writing the graphical interface using code only.
- Designing the graphical interface using the “Glade” program; which is RAD tool to design GTK+ interfaces easily, Glade generates the GUI as a XML file which can be used with any programming language to build the GUI, after exporting the GUI’s XML file, we’ll be able to link the XML file with our program to do the jobs we want.
We’ll explain both ways in short.
The Code-Only Way
Writing the GUI using code only can be little bit hard for noob programmer’s and very time-wasting, but using it, we can create very functional GUIs for our programs, more than those we create using some tools like Glade.
Let’s take the following example.
#!/usr/bin/python # -*- coding: utf-8 -*- from gi.repository import Gtk class ourwindow(Gtk.Window): def __init__(self): Gtk.Window.__init__(self, title="My Hello World Program") Gtk.Window.set_default_size(self, 400,325) Gtk.Window.set_position(self, Gtk.WindowPosition.CENTER) button1 = Gtk.Button("Hello, World!") button1.connect("clicked", self.whenbutton1_clicked) self.add(button1) def whenbutton1_clicked(self, button): print "Hello, World!" window = ourwindow() window.connect("delete-event", Gtk.main_quit) window.show_all() Gtk.main()
Copy the above code, paste it in a “test.py” file and set 755 permission on the test.py file and run the file later using “./test.py”, that’s what you will get.
# nano test.py # chmod 755 test.py # ./test.py
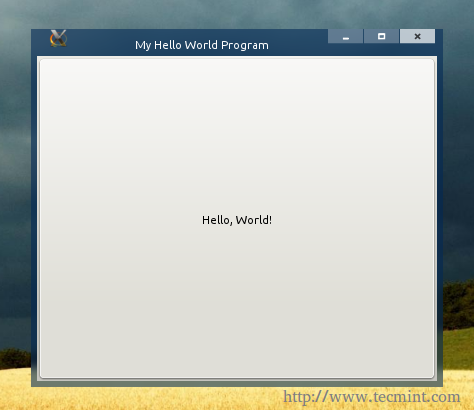
By clicking the button, you see the “Hello, World!” sentence printed out in the terminal:

Let me explain the code in detailed explanation.
- #!/usr/bin/python: The default path for the Python interpreter (version 2.7 in most cases), this line must be the first line in every Python file.
- # -*- coding: utf-8 -*-: Here we set the default coding for the file, UTF-8 is the best if you want to support non-English languages, leave it like that.
- from gi.repository import Gtk: Here we are importing the GTK 3 library to use it in our program.
- Class ourwindow(Gtk.Window): Here we are creating a new class, which is called “ourwindow”, we are also setting the class object type to a “Gtk.Window”.
- def __init__(self): Nothing new, we’re defining the main window components here.
- Gtk.Window.__init__(self, title=”My Hello World Program”): We’re using this line to set the “My Hello World Program” title to “ourwindow” window, you may change the title if you like.
- Gtk.Window.set_default_size(self, 400,325): I don’t think that this line need explanation, here we’re setting the default width and height for our window.
- Gtk.Window.set_position(self, Gtk.WindowPosition.CENTER): Using this line, we’ll be able to set the default position for the window, in this case, we set it to the center using the “Gtk.WindowPosition.CENTER” parameter, if you want, you can change it to “Gtk.WindowPosition.MOUSE” to open the window on the mouse pointer position.
- button1 = Gtk.Button(“Hello, World!”): We created a new Gtk.Button, and we called it “button1”, the default text for the button is “Hello, World!”, you may create any Gtk widget if you want.
- button1.connect(“clicked”, self.whenbutton1_clicked): Here we’re linking the “clicked” signal with the “whenbutton1_clicked” action, so that when the button is clicked, the “whenbutton1_clicked” action is activated.
- self.add(button1): If we want our Gtk widgets to appear, we have to add them to the default window, this simple line adds the “button1” widget to the window, it’s very necessary to do this.
- def whenbutton1_clicked(self, button): Now we’re defining the “whenbutton1_clicked” action here, we’re defining what’s going to happen when the “button1” widget is clicked, the “(self, button)” parameter is important in order to specific the signal parent object type.
- print “Hello, World!”: I don’t have to explain more here.
- window = ourwindow(): We have to create a new global variable and set it to ourwindow() class so that we can call it later using the GTK+ library.
- window.connect(“delete-event”, Gtk.main_quit): Now we’re connecting the “delete-event” signal with the “Gtk.main_quit” action, this is important in order to delete all the widgets after we close our program window automatically.
- window.show_all(): Showing the window.
- Gtk.main(): Running the Gtk library.
That’s it, easy isn’t? And very functional if we want to create some large applications. For more information about creating GTK+ interfaces using the code-only way, you may visit the official documentation website at:
The Glade Designer Way
Like I said in the beginning of the article, Glade is a very easy tool to create the interfaces we need for our programs, it’s very famous among developers and many great applications interfaces were created using it. This way is called “Rapid applications development”.
You have to install Glade in order to start using it, on Debian/Ubuntu/Mint run:
$ sudo apt-get install glade
On RedHat/Fedora/CentOS, run:
# yum install glade
After you download and install the program, and after you run it, you will see the available Gtk widgets on the left, click on the “window” widget in order to create a new window.
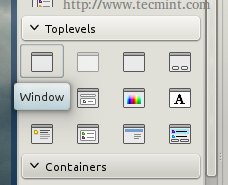
You will notice that a new empty window is created.
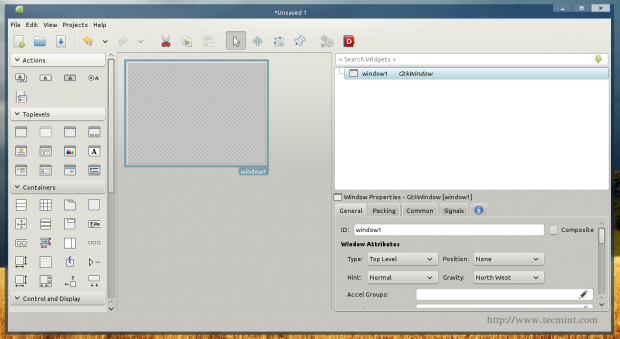
You can now add some widgets to it, on the left toolbar, click on the “button” widget, and click on the empty window in order to add the button to the window.
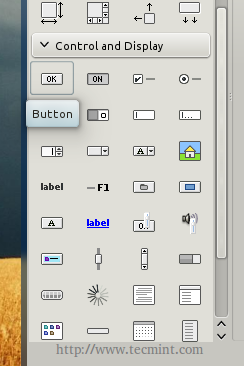
You will notice that the button ID is “button1”, now refer to the Signals tab in the right toolbar, and search for the “clicked” signal and enter “button1_clicked” under it.
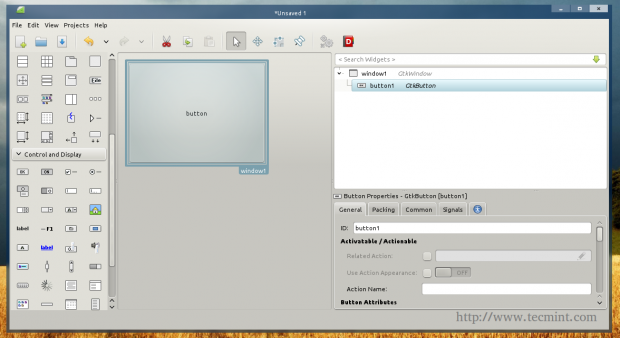
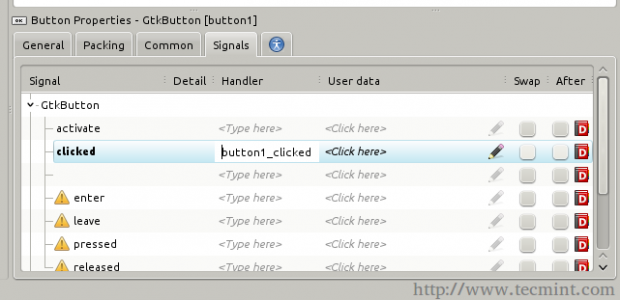
Now that we’ve created our GUI, let’s export it. Click on the “File” menu and choose “Save”, save the file in your home directory in the name “myprogram.glade” and exit.
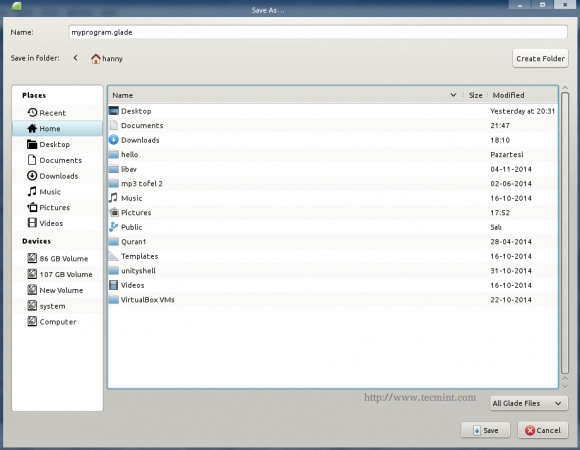
Now, create a new “test.py” file, and enter the following code inside it.
#!/usr/bin/python # -*- coding: utf-8 -*- from gi.repository import Gtk class Handler: def button_1clicked(self, button): print "Hello, World!" builder = Gtk.Builder() builder.add_from_file("myprogram.glade") builder.connect_signals(Handler()) ournewbutton = builder.get_object("button1") ournewbutton.set_label("Hello, World!") window = builder.get_object("window1") window.connect("delete-event", Gtk.main_quit) window.show_all() Gtk.main()
Save the file, give it 755 permissions like before, and run it using “./test.py”, and that’s what you will get.
# nano test.py # chmod 755 test.py # ./test.py
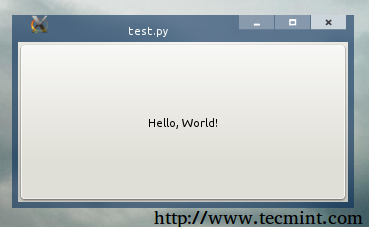
Click on the button, and you will notice that the “Hello, World!” sentence is printed in the terminal.
Now let’s explain the new things:
- class Handler: Here we’re creating a class called “Handler” which will include the the definitions for the actions & signals, we create for the GUI.
- builder = Gtk.Builder(): We created a new global variable called “builder” which is a Gtk.Builder widget, this is important in order to import the .glade file.
- builder.add_from_file(“myprogram.glade”): Here we’re importing the “myprogram.glade” file to use it as a default GUI for our program.
- builder.connect_signals(Handler()): This line connects the .glade file with the handler class, so that the actions and signals that we define under the “Handler” class work fine when we run the program.
- ournewbutton = builder.get_object(“button1”): Now we’re importing the “button1” object from the .glade file, we’re also passing it to the global variable “ournewbutton” to use it later in our program.
- ournewbutton.set_label(“Hello, World!”): We used the “set.label” method to set the default button text to the “Hello, World!” sentence.
- window = builder.get_object(“window1”): Here we called the “window1” object from the .glade file in order to show it later in the program.
And that’s it! You have successfully created your first program under Linux!
Of course there are a lot more complicated things to do in order to create a real application that does something, that’s why I recommend you to take a look into the GTK+ documentation and GObject API at:
Have you developed any application before under the Linux desktop? What programming language and tools have used to do it? What do you think about creating applications using Python & GTK 3?
I appreciate the start of using glade. I found that there were few syntax errors in the “Glade the Designer Way” causing the program to crash.
I found that:
Handler “button_1click” should be “button1_click”
The file “test.py” should read the following:
Need some help with that snake thing, I don’t I like C++.
I’m finding this very interesting. I used to program years ago – mainly in BASIC, and small dalliances with C & COBOL (a very elegant language, essentially like writing English sentences).
I become fed up with Windows 10, which has basically ruined my HP Pavilion dv7 (the Windows 7 it originally came with ran far better), so I bought a second hand MacBook Pro, which I love and is perfect for all my everyday uses, especially media – I’m a keen photographer and also getting into film-making. I would have bought a new MacBook, but if I’m honest I couldn’t afford it, and they’ve stopped doing the 17″ screens anyway – big mistake, Apple – but I digress.
Being loathed to get rid of the HP, whose hardware is of a similarly high spec to the MacBook, I’ve decided to use it for a very different purpose, effectively restoring my HP with Linux Mint Tara, which looks like a really elegant system, and and using it to get back into programming with this impressive Python language, so I basically wanted to say thank you to the author for writing these tutorials, and to ask the community for any tips you may have for someone who, while reasonably well-versed with computers generally, is very much out of date on the nuts & bolts side, and basically a beginner where Linux & Python are concerned.
Thank you in advance for any helpful replies.
Nice indentation there. Must be some real code experts on this site
BaCon (Basic to C Convertor) is an easy programming tool.
Thank you Hanny and tecmint.com for publishing this wonderful, step by step, instructional article, covering all aspects of GUI design, including packaging. In fact, I found the “Desktop Recorder” Application in part 3, so useful, that I developed a couple of my own applications, on similar lines and even packaged it for Debian as well.
The entire project ( including packaging for Debian ) that I did, is shared for the benefit of viewers here : https://sourceforge.net/projects/python-glade-application-desig/
It can resolve most of the issues reported here and also give tips on coding and packaging as well. If you need any more inputs, a simple Google Search will do.
Also, tips on Glade File Design can be seen here : https://youtu.be/86QppCSRNkI and tips on icon design here : https://youtu.be/arPVw8VWM_0
P.S. For those interested, my other project source is shared here : https://sourceforge.net/projects/python-glade-application-de-ii/
P.S. While Porting the above sources from Python 2.7 to Python 3.5, I realized a few new things :
1. Support for tabs as an indentation method, has been removed
2. 4 spaces is the preferred method of indentation
3. Modules like subprocess, stringio etc. has undergone changes.
I have upgraded the sources mentioned above, to reflect these new changes. Please download them again to get the latest version.
After gaining confidence, in Coding & Packaging, using Hanny’s Examples & the Sources Shared above, Viewers may try developing a few simple Apps, like for eg.
1. A Manpage Creator/Studio App ( Just Google “linux create man page”. You will find examples / structure of a man page. It is just a matter of Adding a Few Special Keywords, while generating/filing a manpage, with your GUI Text Entry’s / Text View’s )
2. A Diary App ( Just a matter of Reading / Writing to Files, based on the Date Picked, on a Calendar Widget )
3. GUI for OS Commands, that can simplify tasks ( Accept Input on Text Entry’s/Views, Process Them, Show Result on Text Entry’s/Views )
4. Apps listed on your Software Manager, to make them even better
If you have developed a Unique, Well Tested ( Across Python Versions i.e. Change First Line of Script ) ( Across Linux Flavours / Versions i.e. Using Virtual Machines ) & Useful App for the World, then you may contact Debian Developers/Mentors ( Sponsors ) on the Debian IRC Channels List (Country Specific also available), who can help you, Publish Your Apps to the World. Also Sponsors Listed on their ftp-master site can be contacted on their Email for further progress.
Note: Utility Apps may require multiple GIT Setups, for Upstream / Downstream Releases.
Good Luck With Your Apps Development / Publishing !
Some info: The #!/usr/bin/python line in the test.py code file above, refers to python 2 series, which is marked for end-of-life in 2020. Hence, viewers can substitute python with python3 in the test.py file, as well as the debian/control and debian/rules files, explained in the part 4 article of this series.
I have upgraded my sources shared above, to reflect all these, and incremented the version numbers to 2.1-1. Future upgrades to them will not carry a notice here.
I am new in developing GUI Application. Which is the best way to develop GUI App in gtk?
Using Glade and writing GUI interface design code
None of your examples work…copying and pasting the code you provided gives me nothing but errors:
“IndentationError: expected an indented block”
PyGIWarning: Gtk was imported without specifying a version first. Use gi.require_version(‘Gtk’, ‘3.0’) before import to ensure that the right version gets loaded.
from gi.repository import Gtk
I would like to see an answer to this issue as I am having the same problem.
typing the code and make sure the indentation is consistent in python.
this https://python-gtk-3-tutorial.readthedocs.io/en/latest/ is a good website for learning pygtk with python 3
Had the same issue. A bit of Google and I found this:
Concerning indention:
Seems like the formatting was just lost. In python tabulation is used instead of bracketing. Colon must be followed by tabulated code, meaning that it’s a part of the block. It’s easy to restore if you can read the code for the slightest.
File “./test.py”, line 5
def __init__(self):
^
IndentationError: expected an indented block
Very useful tutorial. Thank you!
You have a minor syntax error in the first script, try to add parentheses to the print command
Nope, I don’t, I just tested the script and it works perfectly.
There is an error in the script of the builder. The name of the function in the Handler() should be the same as the one in glade.
Instead of buttonclicked, it should be button1_clicked ;)
Thanks for this tuto! Really helpful
Thanks Jimmy ! This error will be fixed.
Greetings.
Thanks a lot !!
it’s exactly what I need
Very cool and usefull content.
I’ve put it already in my task list.
Cheers!!