When it comes to managing and analyzing data, converting files from one format to another is a frequent need. If you are working with CSV (Comma-Separated Values) files in Linux and want to convert them into TSV (Tab-Separated Values) files, you are in the right place because this article will help you with the process to perform the required conversion.
Understanding CSV and TSV
CSV files have been widely used for storing structured data. However, TSV files offer some advantages over CSV files.
While CSV files separate values with commas, TSV files use tabs, which can make data handling easier, especially when dealing with commas within the data itself.
TSV files also tend to be more compatible with various applications and tools commonly used for data processing and analysis.
How to Convert CSV to TSV in Linux
Converting CSV files to TSV files in Linux can be achieved through various methods, which are as follows:
Table of Contents
1. Using awk Command
awk is a powerful text processing tool that allows you to manipulate and transform data efficiently, which is also used to convert a CSV file to a TSV file as shown.
$ awk -F ',' 'BEGIN {OFS="\t"} {$1=$1}1' tecmint.csv > tecmint.tsv $ ls -l tecmint.tsv
Replace tecmint.csv with the actual filename of your CSV file, and tecmint.tsv with the desired filename for the converted TSV file.
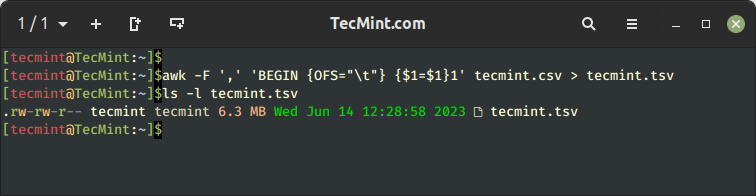
Let’s break down the command:
-F ','
sets the input field separator as a comma, indicating that the input file is in CSV format.BEGIN {OFS="\t"}
sets the output field separator as a tab, specifying that the output file should be in TSV format.{$1=$1}
forces awk to reformat the input fields, using the specified field separators.1
is a common awk pattern that triggers the default action, which is to print the modified record.
2. Using sed Command
The sed command is another powerful tool available in Linux that can be used to convert CSV files to TSV files with ease.
Here is the sed command that you have to execute in the terminal for converting CSV file into a TSV file.
$ sed 's/,/\t/g' tecmint.csv > tecmint.tsv $ ls -l tecmint.tsv
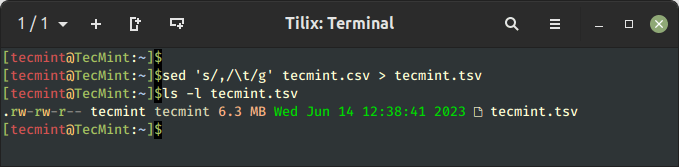
Let’s understand the components of the command:
s/,/\t/g
is the substitution pattern used by sed, which searches for commas(,)
in the input file and replaces them with tabs(\t)
.- input.csv should be replaced with the actual filename of your CSV file.
- output.tsv specifies the desired filename for the converted TSV file. You can choose any name you prefer.
3. Using csvkit Library
The csvkit library provides a convenient and powerful set of command-line tools for working with CSV files in Linux. It offers an easy way to convert CSV files to TSV format.
However, you must first install csvkit on your Linux system from the following command:
$ sudo apt install csvkit [On Debian, Ubuntu and Mint] $ sudo yum install csvkit [On RHEL/CentOS/Fedora and Rocky/AlmaLinux] $ sudo emerge -a sys-apps/csvkit [On Gentoo Linux] $ sudo apk add csvkit [On Alpine Linux] $ sudo pacman -S csvkit [On Arch Linux] $ sudo zypper install csvkit [On OpenSUSE]
Then use the following command with -T
option, which specifies the output delimiter as a tab and converts the CSV file to TSV format.
$ csvformat -T tecmint.csv > tecmint.tsv $ ls -l tecmint.tsv
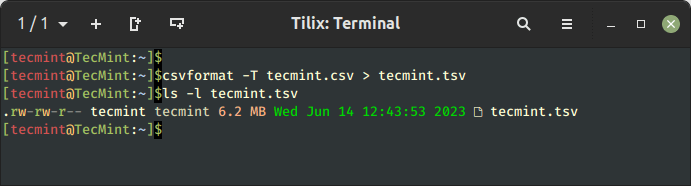
4. Using Python Script
To convert a CSV file to a TSV file in Linux, you can use Python, a versatile programming language that is commonly available in Linux systems. Follow the steps below to use Python for the conversion:
Create a new Python script file in the terminal by running the following command:
$ nano tecmint.py OR $ vi tecmint.py
Then add the following code inside the script file.
import csv csv_file = 'tecmint.csv' tsv_file = 'tecmint.tsv' with open(csv_file, 'r') as input_file, open(tsv_file, 'w') as output_file: csv_reader = csv.reader(input_file) tsv_writer = csv.writer(output_file, delimiter='\t') for row in csv_reader: tsv_writer.writerow(row)
You must replace the CSV file name with your own file name saved in your system and the TSV file name according to your choice.
Then run the Python file using the python3 interpreter:
$ python3 tecmint.py
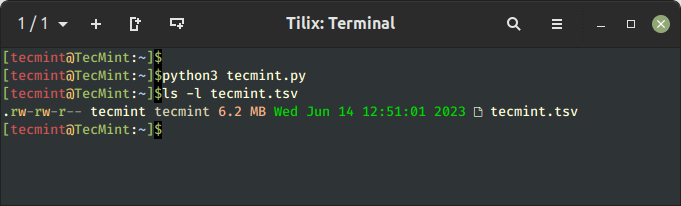
5. Using Perl Script
You can also use Perl programming language in Linux to convert a CSV file to a TSV file. For this purpose, you have to follow the below-given steps:
Create a new Perl script file using the following command:
$ nano tecmint.pl OR $ vi tecmint.pl
Add the following code inside the script file:
#!/usr/bin/perl use strict; use warnings; my $csv_file = 'tecmint.csv'; my $tsv_file = 'tecmint.tsv'; open(my $input_fh, '<', $csv_file) or die "Failed to open $csv_file: $!"; open(my $output_fh, '>', $tsv_file) or die "Failed to create $tsv_file: $!"; while (my $line = <$input_fh>) { chomp $line; my @fields = split(',', $line); my $tsv_line = join("\t", @fields); print $output_fh $tsv_line . "\n"; } close $input_fh; close $output_fh;
Then save the file using CTRL+X
, followed by Y and enter button.
Make the Perl script executable and run the Perl script using the following commands:
$ chmod +x tecmint.pl $ ./tecmint.pl $ ls -l tecmint.tsv
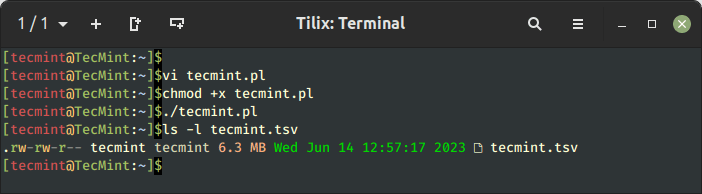
Conclusion
When working with CSV files in Linux and needing to convert them to TSV files, there are several methods available. The article provides step-by-step instructions for using commands like awk and sed, utilizing the csvkit library, using Python, and employing Perl programming language.
Each method offers its own advantages and allows for easy conversion of CSV files to TSV format. By following the provided instructions, users can efficiently perform the required conversion and work with TSV files in their Linux system.
Hey Ravi, thank you for your article.
I believe there missed the most simple way to resolve the topic.
You can just use `tr “,” “\t“`.
For example:
Does it make sense for you?
Simple conversions, but it doesn’t solve the problem mentioned at the beginning of the article.
“While CSV files separate values with commas, TSV files use tabs, which can make data handling easier, especially when dealing with commas within the data itself.”
These methods will convert all commas to tabs, not just the ones that separate the data fields.
@Jose,
To separate the data fields of a CSV file into a TSV (Tab-Separated Values) format using the following awk command.
This command assumes that your input file is named tecmint.csv, and the resulting TSV file will be named tecmint.tsv.