In this Part 3 of Python Data Structure series, we will be discussing what is a dictionary, how it differs from other data structure in python, how to create, delete dictionary objects and methods of dictionary objects.
- Dictionary is a built-in implementation of “Python Data Structure” which is a collection of “Key: Value” pairs.
- Dictionary is created using curly braces with key and value separated by semicolon {Key : Value}.
- Similar to list, dictionaries objects are mutable data type meaning objects can be modified once the dictionary is created.
- The construct of dictionary implementation in python is more generally known as “Associative array”.
- In list or tuples, we can access the items by referencing their index positions because items inside the list are ordered (i.e. Stored in the order they got created). The dictionary objects can be in any order since the items are accessed using its associated “Key”.
- Dictionaries are very useful when we have to store the objects and refer them by name.
- Dictionary “key” object must be a unique and immutable type.
- Dictionary “Key” object can be either string, Integer, Floating values.
- Dictionary “Values” can be of any data type.
Construct Dictionary Object
Dictionary object can be created using curly braces with semicolon separating key and value pair “{Key:value}” or “dict()” constructor method.
To demonstrate, I am going to create a dictionary that will store data about the football team and their playing XI with a position as key and player names as values.
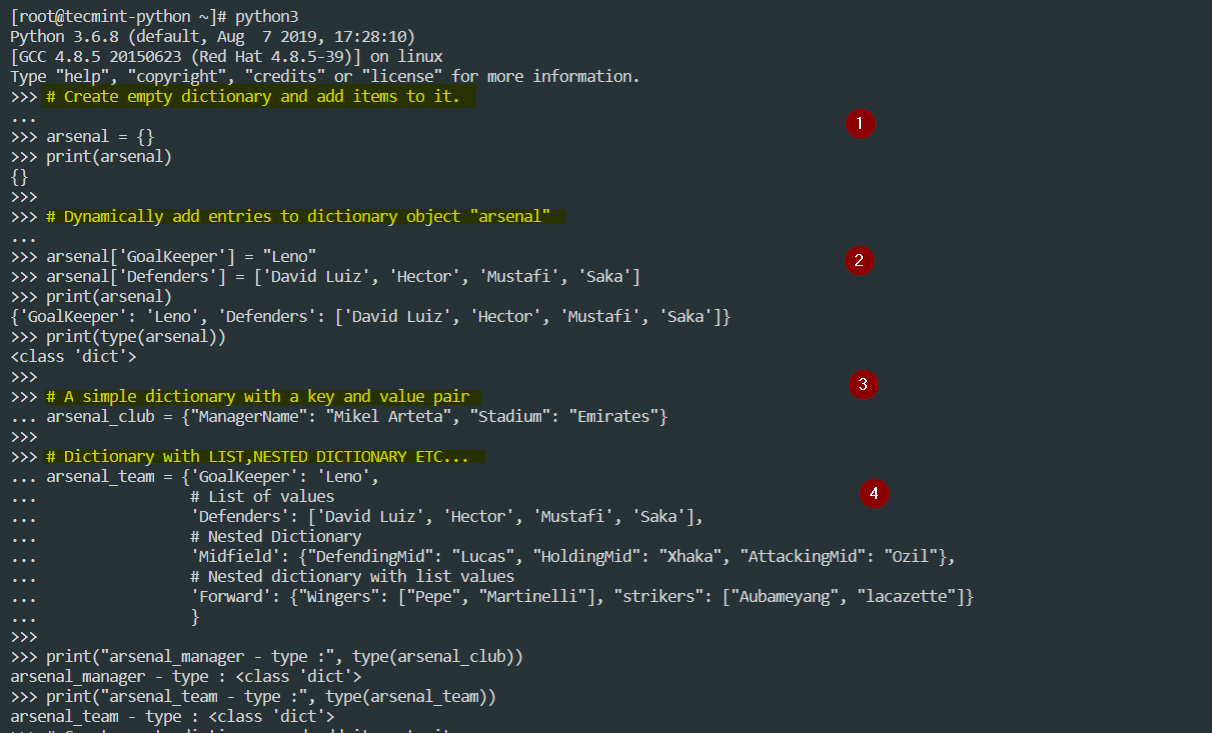
You can use the constructor method dict() to construct a dictionary object.
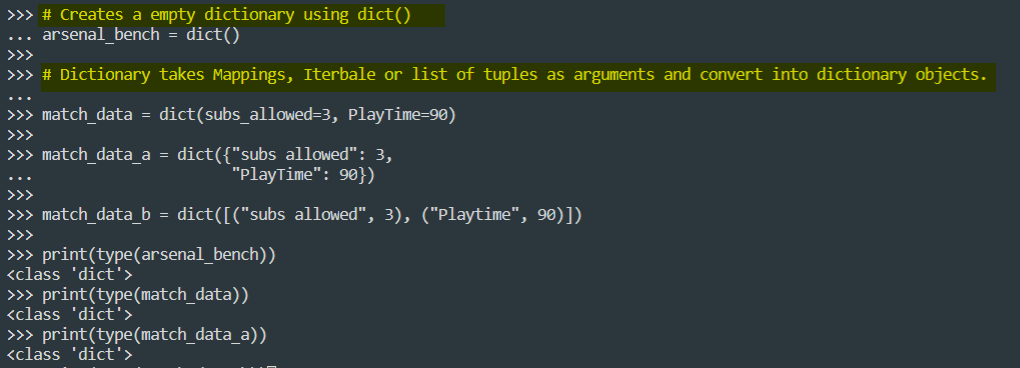
Access Dictonary Object
Dictionary items are accessed by “key” references instead of indexing. It is possible to use indexing if we have any sequence data type (string, list, tuples, etc..) inside the dictionary.
Items can be accessed using dic_object[“key”].
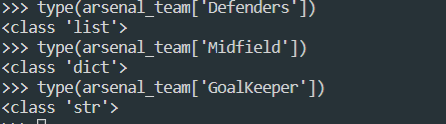
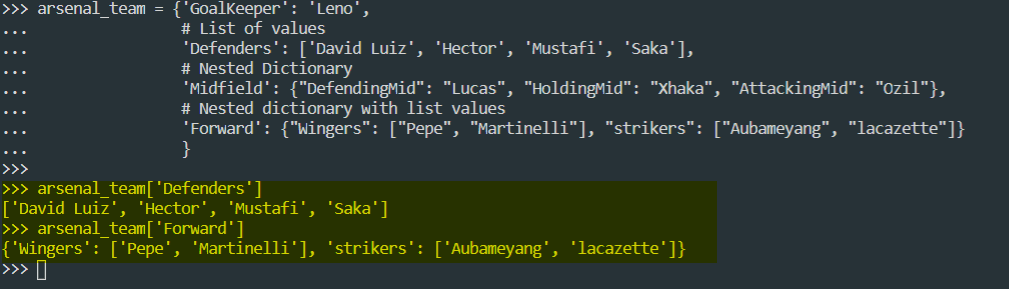
“KeyError” will be raised if you try to access dictionary items with indexing or if you try to access a “key” that is not part of dictionary.
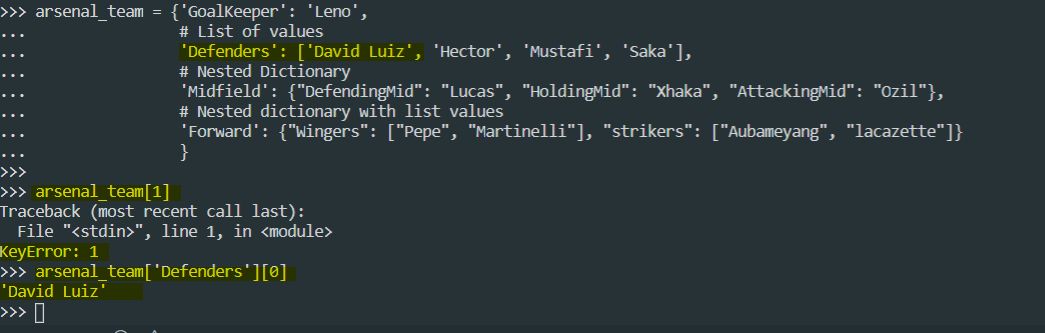
Modify and Delete Dictionary Object
You can modify the existing item or add a new item by directly referencing its key Dictionary_object[“key”] = value. This will update the value if key is available else add new item into the dictionary.
Delete
You can delete a particular value based on its key or delete a key or delete dictionary object from namespace using built-in “del” keyword.
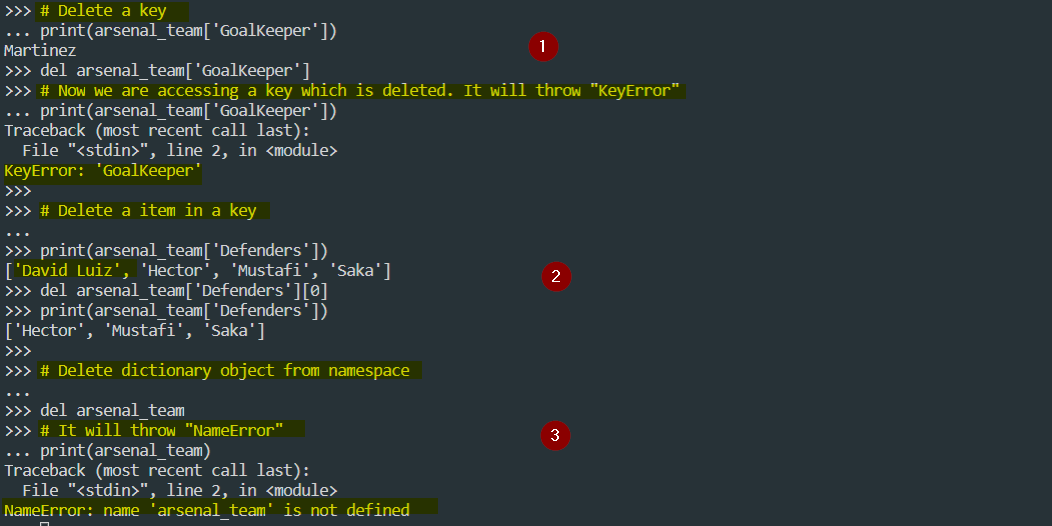
Dictonary Methods
You can use built-in “dir()” function to look up the available methods and attributes for the dictionary object.

clear() – This method will remove all the items from the dictionary object. This method does not take any argument.

Copy() – It will return a shallow copy of a dictionary object. The copy() method doesn’t take any parameters as an argument.

Keys() – This method returns view object for keys available in the dictionary as dictionary key object. This method does not take any argument.

Values() – This method returns a view object for values from the dictionary object. This method takes no argument.

Items() – This method returns a tuple(key,value) pair from the dictionary object.

Setdefault() – This method searches of a given key in a dictionary. If the key is not found in the dictionary then it will be added into the dictionary.
It takes 2 arguments dic.setdefault(key,[,default value]).
The default value is set to None if no value is specified.
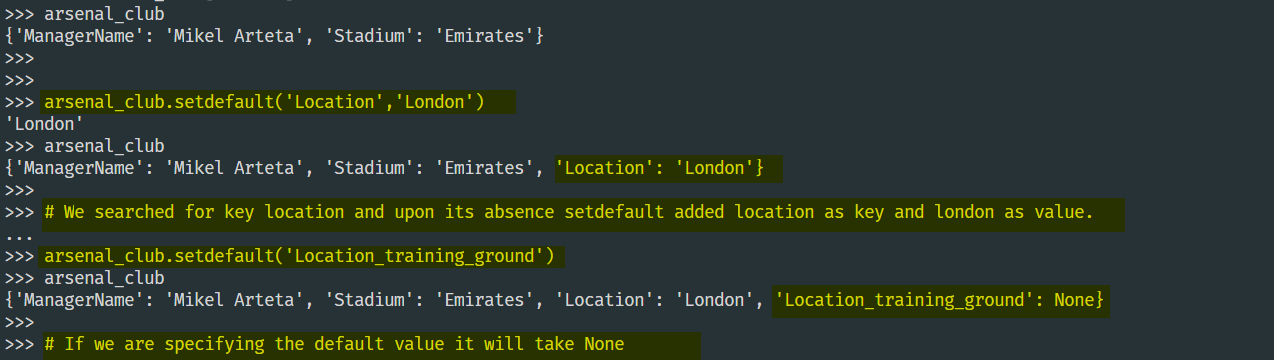
get() – This method returns the value of the specified key if the key is available in a dictionary.
Syntax dict.get(key[, value])
This method takes 2 arguments. First is the input argument that will search for the given key in the dictionary and return the value of the key is found. The second argument will return the value if a key is not found. The default return value is set to “None”.
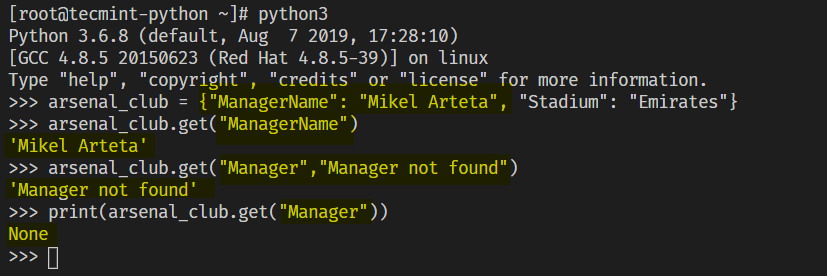
Update() – Update method add items to the dictionary if the key is not in the dictionary. If the key is found that key is updated with the new value. Update method accepts either another dictionary object of k: v pair or iterable object of k: v pair like pair of tuples.
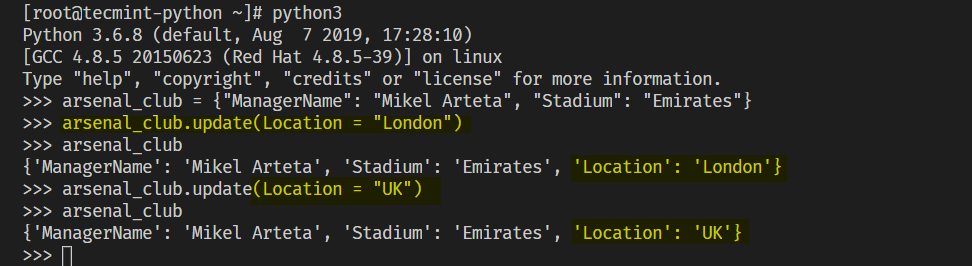
Removing / Deleting Dictionary Object
Pop() – This method removes the value based on the key as input and returns the removed value.
This method accepts two parameters.
- Key – The key to be searched in the dictionary object.
- Default – Return value to be specified if the key is not found in the dictionary.
NOTE If key is not found in the dictionary and if you fail to specify the default value then “KeyError” will be raised.
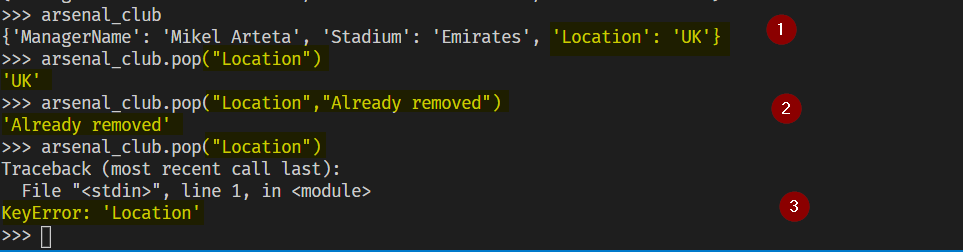
Popitem() – Removes an arbitrary elements from the dictionary object. No argument is accepted and it returns “KeyError” if the dictionary is said to be empty.
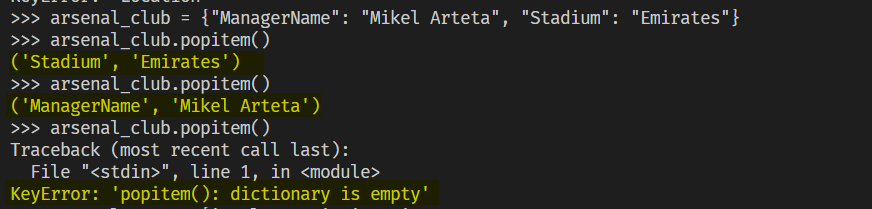
Like list and tuples, we can use a del keyword to remove the items in the dictionary object or remove the dictionary object from the namespace.
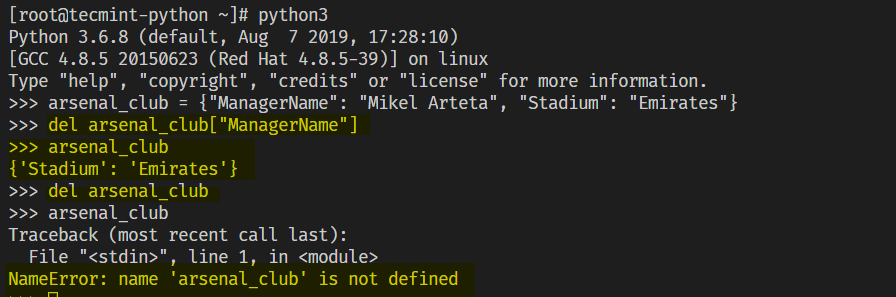
Summary
In this article you have seen what is dictionary and how it differs from other data structures in python. You have also seen how to create, access, modify and delete dictionary objects.
The optimal use case of the dictionary is when we have to store the data based on a name and refer them by its name. In the next article, we will see another type of python built-in data structure “set/Frozenset”. Till then you can read more about dictionaries here.