In this Part 2 of Python Data Structure series, we will be discussing what is a tuple, how it differs from other data structure in python, how to create, delete tuple objects and methods of tuple objects and how tuple differs from the list.
- Python tuples are similar to list data structure but the main difference between list and tuple is, the list is mutable type while tuples are immutable type.
- Tuples support indexing (both positive and negative indexing) and slicing operations.
- Tuples, in general, will be used to store heterogeneous data.
- Compared to list iterating over tuple is fast.
- Tuples can be used as a “key” to dictionary objects since they are hashable.
- We can also store mutable data type inside the tuple like list, set, etc.
- Elements of tuples cannot be modified unless the element is of mutable type.
- Tuples are represented using parenthesis
"()"
.
Construct Tuple Object
Similar to list tuple also has 2 ways of constructing the object.
- Tuple constructor method “tuple()”.
- Parenthesis with values separated by a comma.
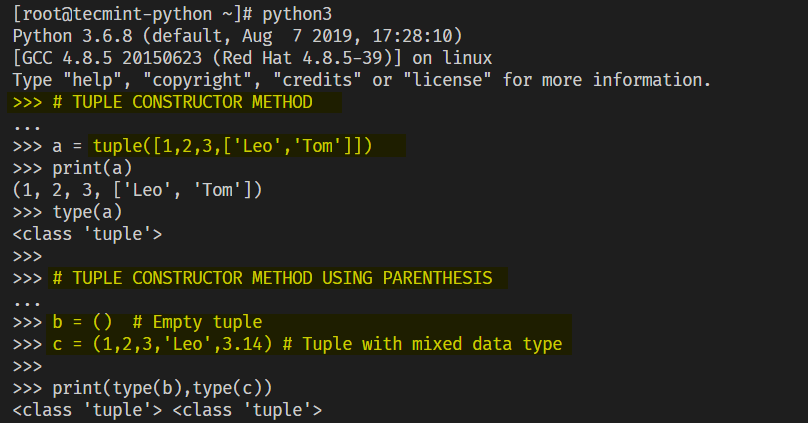
NOTE: You can create empty tuple or tuple with many values, but when you are creating tuple with one value you should add a trailing comma to it otherwise it will not be considered as a tuple object.

You can also create tuple without parenthesis by assigning multiple values to a variable separated by commas and that will be converted to tuple object. This is called as tuple packing.

Tuple Indexing and Slicing
Similar to the list, tuple also supports indexing and slicing operation.
Each item in the tuple is assigned to an index position starting from (0) and negative index position starting from (-1). We can access the index position to get the value or even we can update the tuple item if it is only of mutable types like a list or set.
We can also use slicing to access the items in the list. Slicing allows us to access a range of items by defining the starting, ending, step parameters.
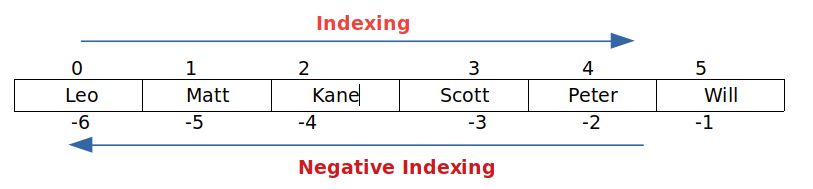
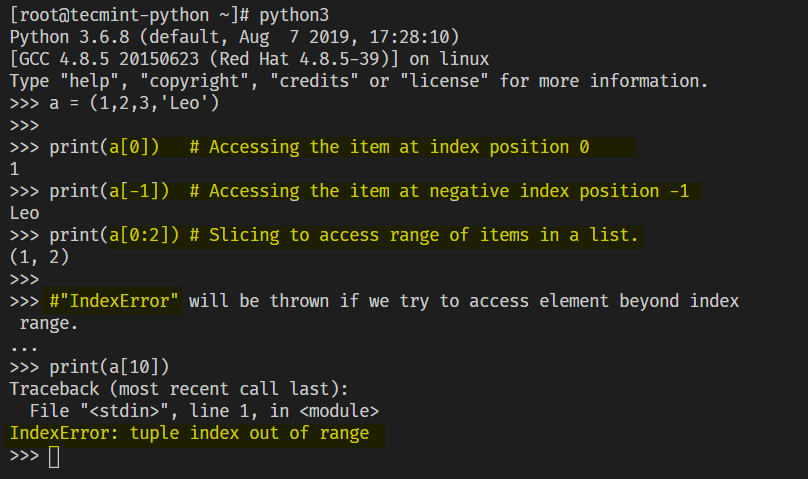
Tuple being an immutable type you cannot modify or remove elements from tuple but we can modify or delete a mutable element that is present inside a tuple.
Consider the example:
b = (1,2,3,'Leo',[12,13,14],(1.1,2.2))
There is a mutable object list inside tuple b at index 4. Now we can modify or delete the elements of this list.

Tuple Methods
Use built-in “dir()”
function to access the methods and attributes for tuple objects.

count(x) method – Returns the number of times x is present in the tuple.
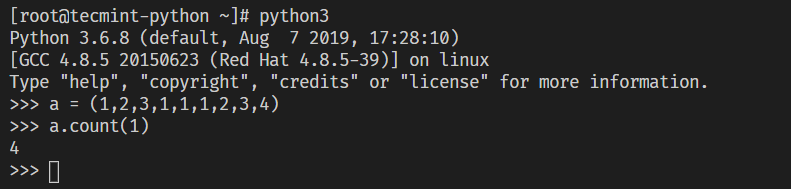
Index(x) method – Returns the first index position of x.
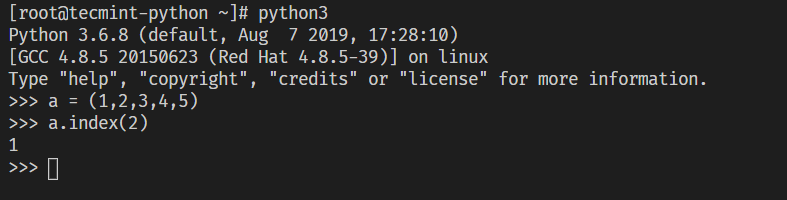
Similar to the list we can combine two tuple objects into a single object using “+”
operator.

Removing and Deleting Tuple Object
Tuple being an immutable type we cannot remove elements from it. We can delete the tuple object from the namespace using the built-in keyword “del”
.
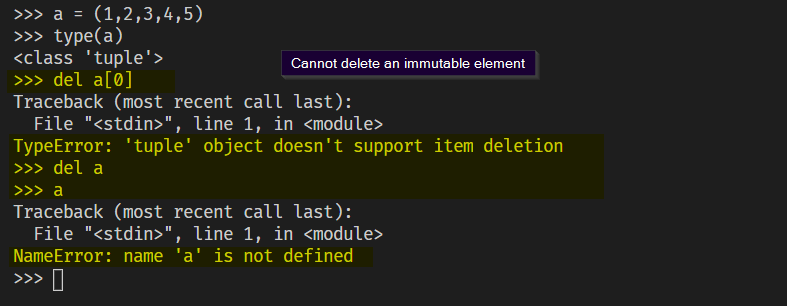
Summary
In this article, you have seen what is a tuple, how tuple is constructed, how to use indexing and slicing operations, tuple methods, etc. Tuple being an immutable type can be used as “key” to dictionary objects. Iterating through a tuple is faster compared to the list. It is best to use tuple when we have our data to remain constant throughout our program.
In the next article, we will be taking a look at another built-in data structure dictionary. Till then, you can read more about Tuples here.