In this Part 4 of Python Data Structure series, we will be discussing what is a set, how it differs from other data structure in python, how to create set objects, delete set objects and methods of set objects.
- A set object is an unordered collection of distinct hashable objects.
- Set automatically removes duplicate items from the object.
- Since set objects are unordered, no indexing and slicing operation is supported.
There are currently two built-in set types.
- set – Since it is mutable, it has no hash value and cannot be used as either a dictionary key or as an element of another set.
- frozenset – Immutable and hashable – its contents cannot be altered after it is created; it can, therefore, be used as a dictionary key or as an element of another set.
Construct Set Object
Create a set using constructor method “set()” or using curly braces with comma separating the elements “{a,b,c}”.
NOTE: you cannot construct a set object through empty braces as it will create dictionary object.
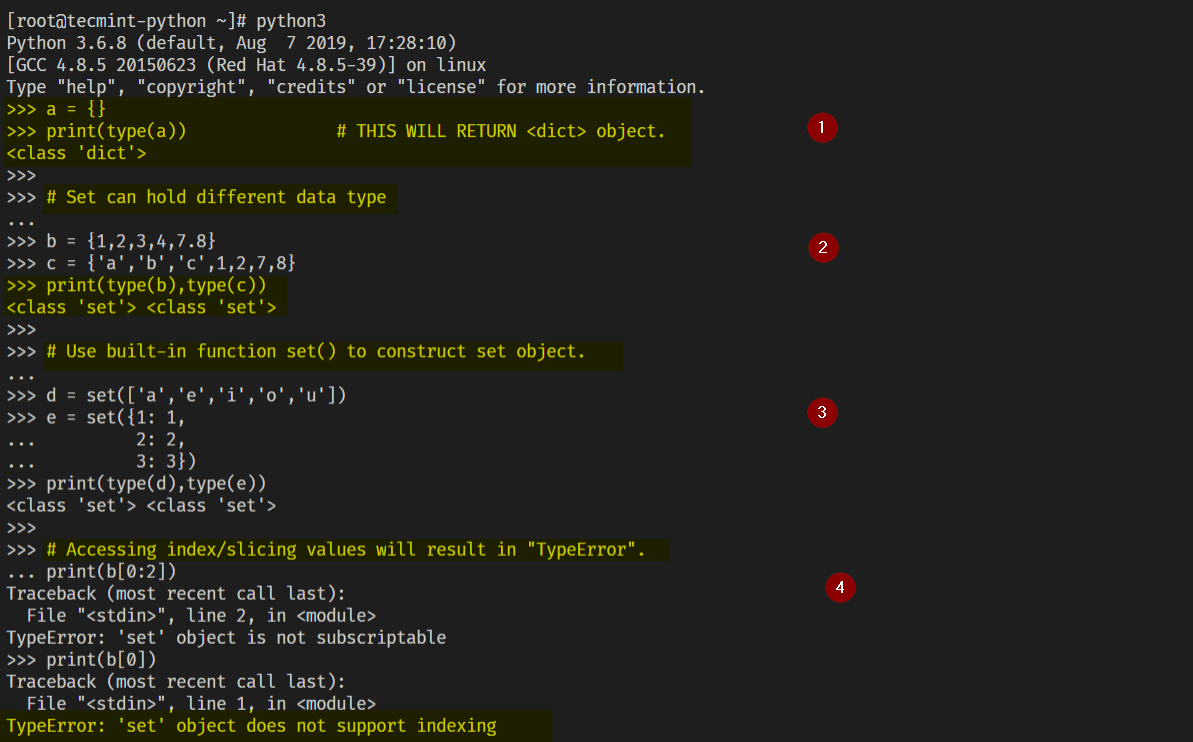
Set Methods
Use built-in “dir()” function to list the available set methods and attributes.

Add Elements to Set Object
As already stated, set is a mutable type. You can add, delete, update your set object once it is created.
Let us talk about two set method add and update.
- add(elem) method – This method adds a single element to a set object.
- update(*others) method – This method adds multiple elements to a set object. You can pass mutable/immutable objects as an argument in the update method.
NOTE: Duplicates will be automatically removed.
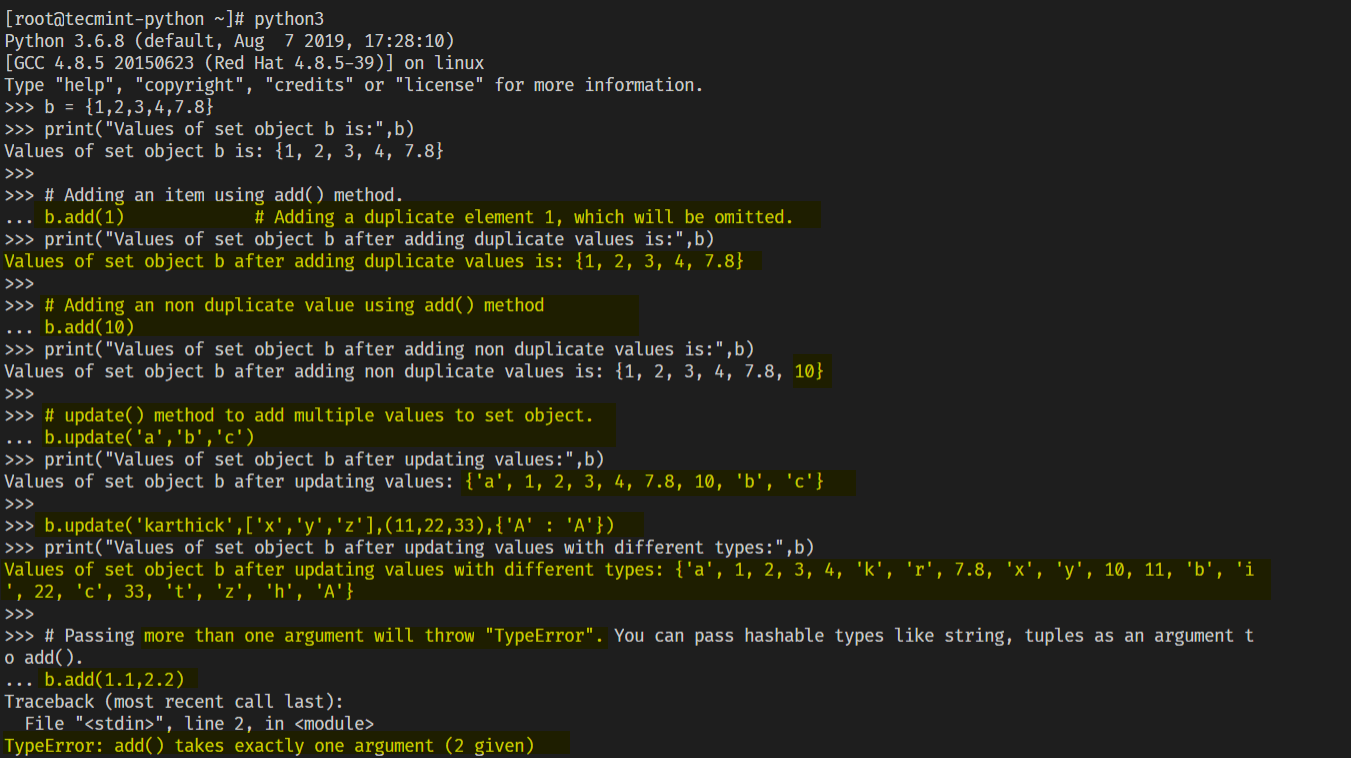
Remove / Clear Elements From a Set Object
As you have seen previously in other data structure topic (list, tuples, dictionary), for set also you can use built-in keyword “del” to delete the set object from namespace (i.e Memory).
Below are the methods for set objects to remove elements.
- clear() – Will clear all the elements making the set empty. This clear() method is available in other data structures providing the same functionality.
- pop() – Removes arbitrary elements.
- discard(elem) – If the item is not found in the set object then “discard()” method will not raise any error.
- remove(elem) – Same like “discard()” method but it will raise KeyError when an item is not found.
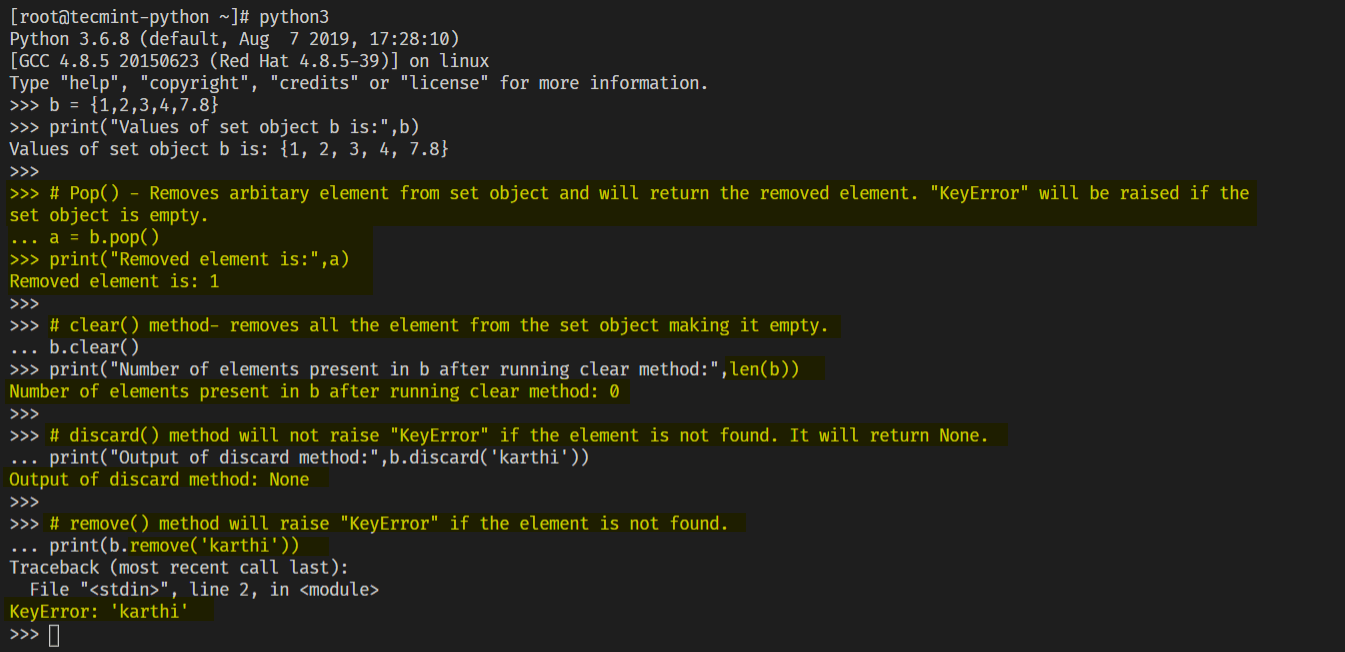
Set Operations
Set provides methods to perform mathematical operations such as intersection, union, difference, and symmetric difference. Remember “Venn diagram” from your highs school days?
We will take a look at the below methods on how mathematical operations are performed.
- union
- intersection
- intersection_update
- symmetric_difference
- symmetric_difference_update
- difference
- difference_update
- isdisjoint
- issubset
- issuperset
Union, Intersectio, Difference, Symmetric_Difference
- union(*other) – Return a new set with elements from the set and all others.
- intersection(*other) – Return a new set with elements common to the set and all others.
- difference(*others) – Return a new set with elements in the set that are not in the others.
- symmetric_difference(other) – Return a new set with elements in either the set or other but not both.
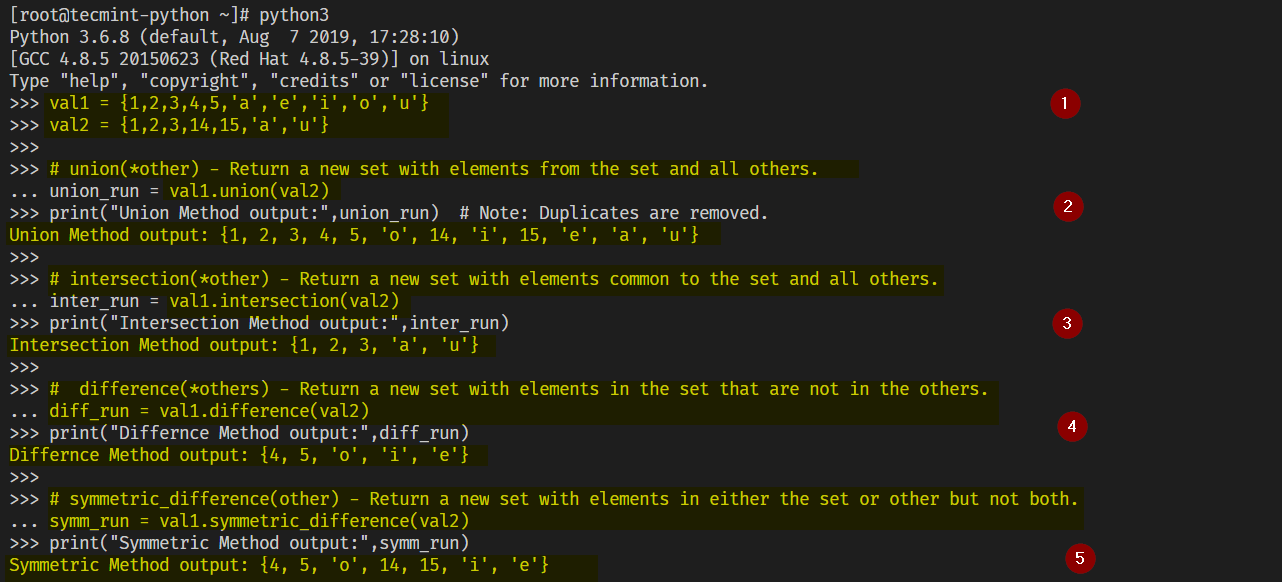
Intersection_Update
intersection_update(*others) – Update the set, keeping only elements found in it and all others.
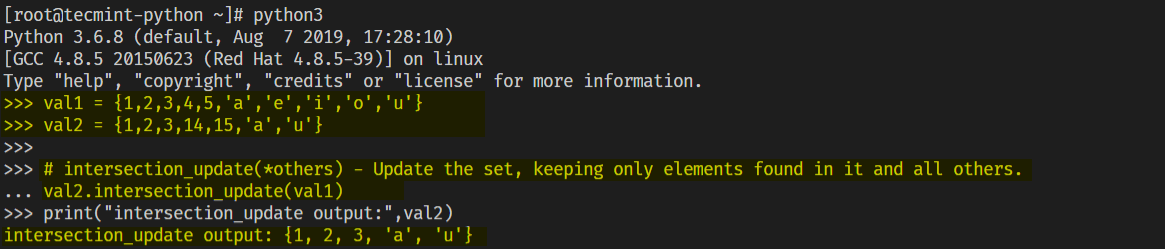
Difference Update
difference_update(*others) – Update the set, keeping only elements found in it and all others.

Symmetric_Difference_Update
symmetric_difference_update(other) – Update the set, keeping only elements found in either set, but not in both.

Isdisjoint, Issubset, Issuperset
- isdisjoint(other) – Return True if the set has no elements in common with other. Sets are disjoint if and only if their intersection is the empty set.
- issubset() – Test whether every element in the set is in another.
- issuperset() – Test whether every element in the other is in the set.
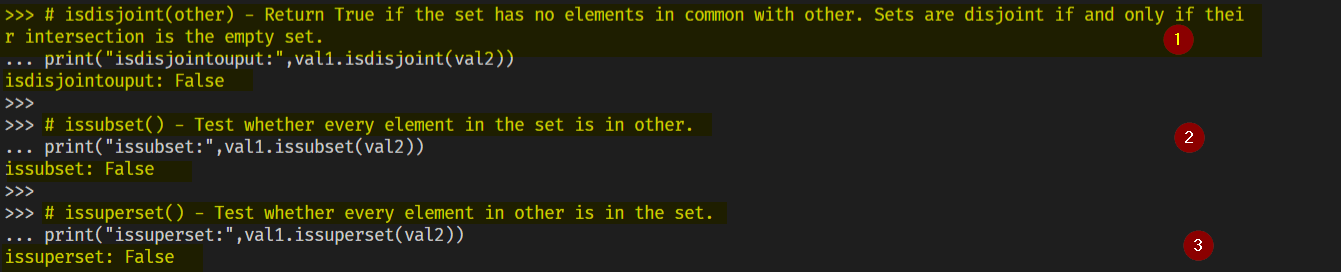
Copy() Method
You can create an identical copy of existing set object using copy() method. This method is also available for other data structure types like list, dictionary etc…
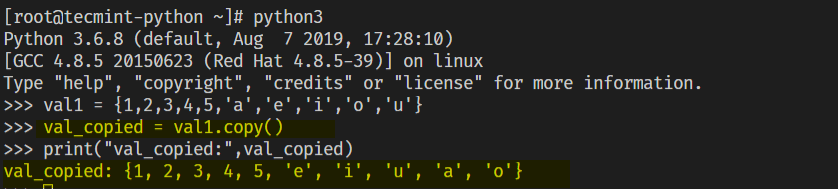
Delete the set object from the namespace using a built-in “del” keyword.
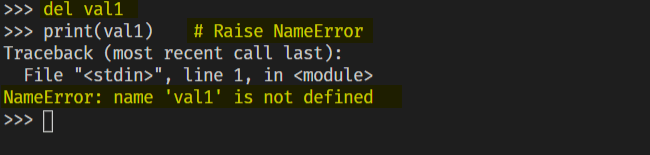
Frozen Set
- Frozen set is immutable type. Once constructed you cannot add, remove or update elements from the list.
- Frozen set being immutable are hashable, can be used as a “key” for dictionaries or elements for another set object.
- Frozen set is constructed using “frozenset()” function.
- Frozen set provides same set of methods in comparison to “set” like union(), intersection, copy(), isdisjoint() etc.
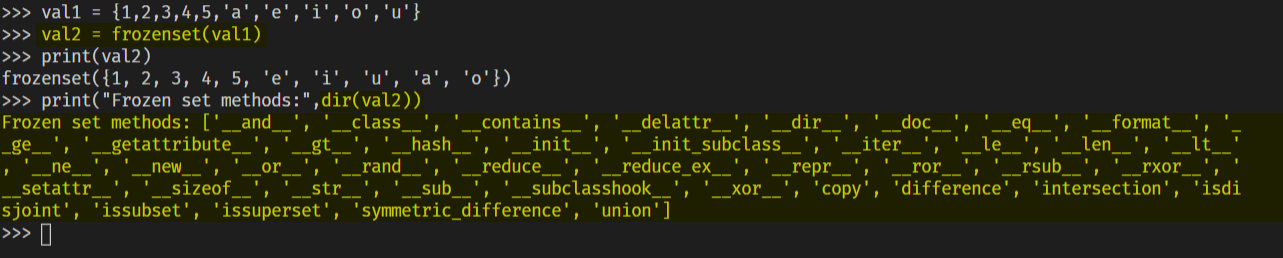
Summary
In this article you have seen what is set, difference between set and frozen set, how to create and access the set elements, set methods etc…