You People would be feeling comfortable, understanding Shell Scripts and writing them fluently, as per your need. This is the last post of this tutorial series, where we will be carrying out a bit complex Mathematical Operations using scripting language. The last four articles of Shell Scripting series which are chronologically.
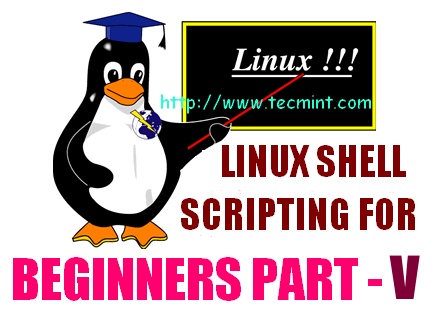
- Understand Basic Linux Shell Scripting Language Tips – Part I
- 5 Shell Scripts for Linux Newbies to Learn Shell Programming – Part II
- Sailing Through The World of Linux BASH Scripting – Part III
- Mathematical Aspect of Linux Shell Programming – Part IV
Lets start with Fibonacci Series
A pattern of numbers where each number is the sum of two preceding numbers. The series is 0, 1, 1, 2, 3, 5, 8…… By definition, the first two numbers in the Fibonccai sequence are 0 and 1.
Script 1: Fibonacci.sh
#!/bin/bash echo "How many numbers do you want of Fibonacci series ?" read total x=0 y=1 i=2 echo "Fibonacci Series up to $total terms :: " echo "$x" echo "$y" while [ $i -lt $total ] do i=`expr $i + 1 ` z=`expr $x + $y ` echo "$z" x=$y y=$z done
Sample Output
[root@tecmint ~]# chmod 755 Fibonacci.sh [root@tecmint ~]# ./Fibonacci.sh How many numbers do you want of Fibonacci series ? 10 Fibonacci Series up to 10 terms :: 0 1 1 2 3 5 8 13 21 34
You are Familiar with the fact that computer understand only in the Binary Format, i.e., ‘0‘ and ‘1‘ and most of us have enjoyed learning the conversion of Decimal to Binary. How about writing a simple script for this complex operation.
Script 2: Decimal2Binary.sh
#!/bin/bash for ((i=32;i>=0;i--)); do r=$(( 2**$i)) Probablity+=( $r ) done [[ $# -eq 0 ]] &echo -en "Decimal\t\tBinary\n" for input_int in $@; do s=0 test ${#input_int} -gt 11 &printf "%-10s\t" "$input_int" for n in ${Probablity[@]}; do if [[ $input_int -lt ${n} ]]; then [[ $s = 1 ]] && printf "%d" 0 else printf "%d" 1 ; s=1 input_int=$(( $input_int - ${n} )) fi done echo -e done
Sample Output
[root@tecmint ~]# chmod 755 Decimal2Binary.sh [root@tecmint ~]# ./Decimal2Binary.sh 1121 Decimal Binary 1121 10001100001
Note: The above script accept Input at run time, which obviously is an aid.
Well the inbuilt ‘bc‘ command can convert a decimal to binary in a script of single line. Run, at your terminal.
[root@tecmint ~]# echo "obase=2; NUM" | bc
Replace ‘NUM‘ with the number, which you want to convert from Decimal to Binary. For example,
[root@tecmint ~]# echo "obase=2; 121" | bc 1111001
Next we will be writing a script which function just opposite of the above script, Converting Binary Values to Decimal.
Script 3: Binary2Decimal.sh
#!/bin/bash echo "Enter a number :" read Binary if [ $Binary -eq 0 ] then echo "Enter a valid number " else while [ $Binary -ne 0 ] do Bnumber=$Binary Decimal=0 power=1 while [ $Binary -ne 0 ] do rem=$(expr $Binary % 10 ) Decimal=$((Decimal+(rem*power))) power=$((power*2)) Binary=$(expr $Binary / 10) done echo " $Decimal" done fi
Sample Output
[root@tecmint ~]# chmod 755 Binary2Decimal.sh [root@tecmint ~]# ./Binary2Decimal.sh Enter a number : 11 3
Note: The above function can be performed in terminal using ‘bc‘ command as.
[root@tecmint ~]# echo "ibase=2; BINARY" | bc
Replace ‘BINARY‘ with the Binary number, viz.,
[root@tecmint ~]# echo "ibase=2; 11010101" | bc 213
Similarly you can write conversion from octal, hexadecimal to decimal and vice-versa yourself. Accomplishing the above result in terminal using ‘bc‘ command is.
Decimal to Octal
[root@tecmint ~]# echo "obase=8; Decimal" | bc
Decimal to Hexadecimal
[root@tecmint ~]# echo "obase=16; Decimal" | bc
Octal to Decimal
[root@tecmint ~]# echo "ibase=8; Octal" | bc
Hexadecimal to Decimal
[root@tecmint ~]# echo "ibase=16; Hexadecimal" | bc
Binary to Octal
[root@tecmint ~]# echo "ibase=2;obase=8 Binary" | bc
Some of the Common Numeric tests used in shell scripting language with description is.
Test : INTEGER1 -eq INTEGER2 Meaning: INTEGER1 is equal to INTEGER2
Test : INTEGER1 -ge INTEGER2 Meaning: INTEGER1 is greater than or equal to INTEGER2
Test: INTEGER1 -gt INTEGER2 Meaning: INTEGER1 is greater than INTEGER2
Test:INTEGER1 -le INTEGER2 Meaning: INTEGER1 is less than or equal to INTEGER2
Test: INTEGER1 -lt INTEGER2 Meaning: INTEGER1 is less than INTEGER2
Test: INTEGER1 -ne INTEGER2 Meaning: INTEGER1 is not equal to INTEGER2
That’s all for this article, and the article series. This is the last article of Shell Script Series and it does not means that no article on Scripting language will be here again, it only means the shell scripting tutorial is over and whenever we find an interesting topic worth knowing or a query from you people, we will be happy to continue the series from here.
Stay healthy, tuned and connected to Tecmint. Very soon I will be coming with another interesting topic, you people will love to read. Share your valuable thoughts in Comment Section.
You got a typo in script2
@Ian,
Can you point us the typo? so that we can correct it..