The Linux shell, or command-line interface, is a powerful program that enables users to interact with the operating system via text-based commands.
Understanding the basics of the Linux shell and shell scripting can greatly enhance your efficiency and control over your system.
In this guide, we’ll explore key concepts and provide tips for both beginners and those looking to delve into basic shell scripting.
Understanding Linux Shell
The shell is a command-line program that interprets user commands and translates them into actions that the operating system can perform. It acts as an intermediary between the user and the Linux kernel, enabling users to control and manipulate the system through commands.
- Shell – A command-line interpreter that connects a user to the operating system, allowing the execution of commands or the creation of text scripts.
- Process – Any task that a user runs in the system is called a process. A process is a bit more complex than just a task.
- File – A file resides on the hard disk (HDD) and contains data owned by a user.
- X-Windows (X11) – A mode of Linux where the screen (monitor) can be split into small “parts” called windows, allowing a user to perform several tasks simultaneously, switch from one task to another easily, and view graphics in a visually appealing way.
- Terminal – A monitor that has only the capability of displaying text, without graphics or with very basic graphics.
- Session – The time between logging on and logging out of the system.
Types of Linux Shells
Linux supports several types of shells, with Bash (Bourne Again Shell) being the most prevalent. Other notable shells include Zsh (Z Shell), Fish, and Dash.
Each shell has its features and syntax, catering to different user preferences and needs.
- Bash – It is the default shell for most Linux distributions and is widely used due to its versatility and powerful scripting capabilities.
- Zsh – It is known for its enhanced features and improved user interface. It incorporates features from other shells, including Bash and Korn.
- Fish – It is designed to be user-friendly with a focus on simplicity and discoverability by providing syntax highlighting and auto-suggestions for commands.
- Dash – It is a lightweight shell designed for efficiency that is often used as the default system shell on minimalistic Linux distributions.
- Ksh – It is a powerful shell with a focus on both interactive use and scripting that incorporates features from the Bourne shell (sh) and the C shell (csh).
- Csh – It is designed with a syntax resembling the C programming language, which is known for its interactive features and scripting capabilities.
Basic Commands
There are thousands of commands for command-line users. How about remembering all of them? Hmm! Simply, you cannot. The real power of a computer is to simplify your work. To ease your work, you need to automate processes, and hence, you need scripts.
Scripts are collections of commands stored in a file. The shell can read this file and act on the commands as if they were typed at the keyboard. The shell also provides a variety of useful programming features to make scripts truly powerful.
Basics of Shell Programming
Shell programming involves creating scripts that utilize the capabilities of a shell, such as Bash, to automate tasks and execute commands. Understanding the basics of shell programming is essential for efficient and customized usage of the command-line interface.
Shebang (#!) Line
Every shell script begins with a shebang line specifying the interpreter to execute the script.
For Bash scripts, it is commonly #!/bin/bash
.
#!/bin/bash
Comments
Use #
to add comments to your script. Comments are for human readability and are ignored by the shell.
# This is a comment
Variables
Variables store data in a script. Use the assignment operator (=)
without spaces to assign values to variables.
greeting="Hello, Shell!"
User Input
Use the read command to obtain user input during script execution.
read -p "Enter your name: " username
Echo
The echo command is used to print output to the terminal, which is often used for displaying messages or variable values.
echo "Welcome, $username!"
Conditional Statements
Use if, elif, and else for decision-making in scripts.
if [ condition ]; then # code to execute if the condition is true else # code to execute if the condition is false fi
Loops
Use for and while loops for repetitive execution of commands.
for i in {1..5}; do # code to repeat five times done
Functions
Encapsulate code into functions for modularity and reusability.
function greet { echo "Hello, $1!" } greet "John"
Exit Status
Commands return an exit status. Use $?
to check if the last command was successful (exit status 0) or encountered an error.
if [ $? -eq 0 ]; then echo "Command executed successfully." else echo "Error during command execution." fi
File Permissions
When dealing with sensitive operations in scripts, use the chmod command to set appropriate permissions on the file.
chmod +x myscript.sh
About Shell Script
A shell script is simply a text file with a ".sh"
extension that has executable permissions.
Here are some tips to guide you through the process of writing and executing a script:
- Begin your script with a shebang line (#!/bin/bash) to specify the interpreter.
- Add comments to explain the purpose of your script, especially for complex or lengthy code segments.
- Use the echo command to print the “Hello, World!” message to the terminal.
- Before execution, ensure your script has the necessary permissions using the chmod command, e.g., chmod +x script.sh.
“This is how your first shell script looks.”
#!/bin/bash # My first script echo "Hello World!"
Save the above lines on a text file, make it executable, and run it, as described above.
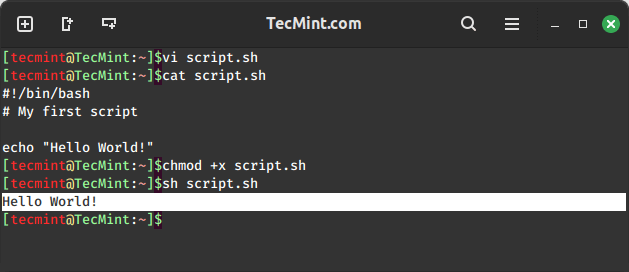
Writing Second Script
Alright, it’s time to move on to the next script, which will display your ‘username‘ and list the currently running processes.
#! /bin/bash echo "Hello $USER" echo "Hey i am" $USER "and will be telling you about the current processes" echo "Running processes List" ps
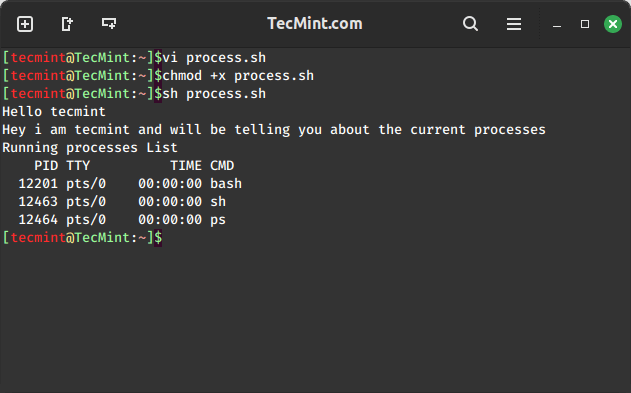
Was this cool? Writing a script is as simple as conceiving an idea and composing a sequence of commands. However, there are some limitations. Shell scripts excel at concise filesystem operations and scripting the integration of existing functionality through filters and command-line tools via pipes.
When your requirements extend beyond, be it in functionality, robustness, performance, efficiency, etc., then transitioning to a more full-featured language is advisable.
If you are already familiar with C/Perl/Python or any other programming language, acquiring proficiency in a scripting language shouldn’t be too challenging.
Writing Third Script
Moving on, let’s write our third and final script for this article. This script functions as an interactive script. Why not try executing this simple yet interactive script yourself and share your experience with us?
#! /bin/bash echo "Hey what's Your First Name?"; read a; echo "welcome Mr./Mrs. $a, would you like to tell us, Your Last Name"; read b; echo "Thanks Mr./Mrs. $a $b for telling us your name"; echo "*******************" echo "Mr./Mrs. $b, it's time to say you good bye"
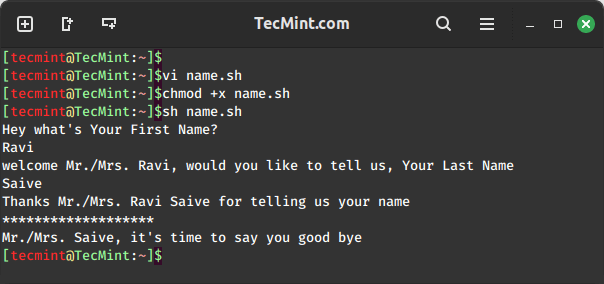
Well, this is not the end. We tried to introduce you to the basics of scripting. In our future articles, we will delve deeper into the topic of scripting languages – a vast and ever-evolving field—to provide a more comprehensive understanding.
Your valuable thoughts in the comments are highly appreciated. Please like and share to help us reach a wider audience. Until then, relax, stay connected, and stay tuned.
Superb article, I am beginner and this tutorial clear my some doubts. thanks for sharing.
Thanks for this article. I am a beginner and your articles are helping me a lot. thanks for your good work.
Please keep posting amazing articles like this.
Thank you very much for your work!
Helped a lot
Thanks man!!
Keep up the good work!!
useful notes did you posted the next in line article on this topic
@Fahad,
Yes, here is the second part of this Linux shell scripting series – https://www.tecmint.com/basic-shell-programming-part-ii/
Useful Notes..Superb!
Good startup for me.
This article helped me to learn basic of scripting. Now I am ready for next step.. :)
Keep it up brother.
very nice tutorial
AWESOME notes
very useful
AWESOME DUDE…
HATS OFF FOR YOUR PATIENCE….
KEEEP GOING LIKE THIS.
thank you!!
Can you tell me the exact meaning of exclamation symbol in [ if ! -f $filename ]
Hey I want to download Linux bible but its not download its show (“Thank you for your interest in Linux Bible– Complimentary Excerpt. We apologize that TradePub.com is no longer able to fulfill requests for this offer.”) I want this book any body send me this book on my email id please …..
thank you
abhishek kushwaha
@Abhishek,
The books is no more available to download, as it is very old and not so useful now due to new changes in Linux, I suggest you to download other similar books.
is there any tools or cmd to see binary file
@Irfan,
You mean to say, how to find binary file in Linux? if yes go for find or grep command to look for same..
https://www.tecmint.com/35-practical-examples-of-linux-find-command/
https://www.tecmint.com/12-practical-examples-of-linux-grep-command/
How that techmint comes in picture in second script
@Narayan,
If you see the second script closely you will see we used $USER variable, that means the current logged in user will display in the output of the script..
Excellent. As a beginner, you ignited the taste and urge to learn more of Shell Scripting. Thanks for your great work.
Very informative to learners!!!
Very Nice…
Good Experience..
Hi,
could you please help me with I want to know if there is any script or setting to lock computer after some idle time in the openLdap domain.
Thank you
This is very good for Starting Shell scrypting tutorial.
Really i am very happy to use first time shell scrypting throught this site.
great , a very simple and easy way to teach.its really amazing.
Well Done
Thanks Avishek for the interesting article, really got me involved. Simple yet effective.
Will follow your posts without fail.
Hello
I have just started the RHEL7 training.I found lots of useful information from this website
It helping me lot. Thanks for sharing knowledge.
Regards
Satish Borkar
Dear Satish,
Good to know our post is helping you.
Keep connected for more such posts.
Hi Avishek,
Thanks for a such information.
I will wait for next part also…
Dear Laxman,
we have already published a lots of post on Shell Scripting.
You may like to look at them here: https://www.tecmint.com/category/bash-shell/
@Avishek
Nice artical for the shell scripting beginners.
Pleased to know Wijaya,
you find the post useful.
Keep connected for more such posts.
a good to start articles for beginners like me.
Thanks sashank,
for your feedback.
Keep connected for more such posts.
I was confused about the first script that you have in this post why you have to read the variables as ‘a’ or ‘b’ and not ‘$a’ but when you output them you write the $ symbol next to them. I think there should be more explanation about this.
Also, another thing that I noticed is that in the first script: you have this:
echo “Hello $USER”
echo “Hey i am” $USER “and will be telling you about the current processes”
why in the first echo you surround $USER with double quotes and not in the second echo?
Why in the third script you have lines ending with ; and some of them that don’t like:
echo “Thanks Mr./Mrs. $a $b for telling us your name”;
echo “*******************”
Thank you
Great website and great tutorial by the way.
@victor1
The first variable is stored in a and the later in b,
we can use the variable the way we want.
read a will wait for the user to get input and will store in the variable a. Similar is the condition with variable b, in the above post.
Hi,
Thanks for this beginners tutorial :) I will continue to explore your blog.
Thanks
Dear Nestor,
Sure! keep connected.
Great.
Thanks for an amazing tutorial dude.
Welcome @vicdeveloper,
keep Connected!
Ji …
Super ji super …
Thank u …
ji…
welcome ji welcome …
Keep Connected!
Excellent tutorial for beginners like me, Avishek. I’m a new Linux user, and I’d like to get started with the terminal commands as soon as I can. This is a good start, I guess. Very well explained. Thanks mate :)
Welcome Akash! we will be expanding this series soon.
Hello thanks for sharing this amazing article. Please, I am having some problem with the third script. when I run the the script, only the first echo statement “what’s your first name” came out. the rest did not show and the shell prompt hang that I had to “cltr c” to bring it back.
I copied and past the above code and give the script executable bit and still having the same issue. please help.
Thanks again
copy and paste the below code as it is, to a file
#! /bin/bash
echo “Hey what’s Your First Name?”;
read a;
echo “welcome Mr./Mrs. $a, would you like to tell us, Your Last Name”;
read b;
echo “Thanks Mr./Mrs. $a $b for telling us your name”;
echo “*******************”
echo “Mr./Mrs. $b, it’s time to say you good bye”
save it.
Make it executable as chmod 755 script_name.
Run as ./script_name
Good work, it helps a lot to the beginners. Thanks a Ton for your efforts. Hope you tecmint will bring more articles of this kind.
Pleased to know that @ Girish!
great work .. nice introductory tutorial for beginners like me. :)
Thanks for the appreciation @ Nitesh
great work,
Thanks for the recognition.
in third script ,
line 3 and 5, i.e: read a; read b;
how script know that a is avishek and b is kumar. ?
echo “Hey what’s Your First Name?”;
read a;
echo “welcome Mr./Mrs. $a, would you like to tell us, Your Last Name”;
read b;
When you type read a, it will show a prompt to accept input from you. the value you provided will be stored in a, if you entered xyjvfdj for a, a will have that value. Similar is the condition for Variable b.
Thank you AvishekKumar ur explanation is very easy and understandable
Welcome @ Pinnaka
nice article man. thank you for this. helped me more on developing my interest in scripting using linux.
Pleased to know that! @ jshnrz
Awesome basic tutorial for beginners
Happy to know it.@ Yuvraj
nice article, Thanks~~
Thanks @ bluedrean
thanks for this nice article….. keep blogging….
Welcome@ Koushik
Thank you very much for this,
i am using ubuntu 13.10 and it took me a while to find how to get started eventually i found vim editor and after that everything was smooth and quite enjoyable
Thank you again
Welcome @ senister
i’ve 2 questions.please try to answer:—–
1. what r enviroment variables and shell variables ?
2. what is name of log file for filesystem check and errors?
Avishek , Thanks once again. Would I need to create variables at the beginning of script. to have user indicate gender vs. and the echo Mr./Mrs. ?
You can create variable at the beginning, as it is an interactive script, if you finds it difficult, you can leave a comment here.
Found your short lesson very helpful. I started thinking right after successfully running the script, and began to wonder if I could create two variables for Mrs and Mr. so that user can specify gender and use that input before name.
Pleased to know it @ vle, Thanks for your feedback. And you can create any number of variable in a script or program.
its very interesting………. it is not as difficult as i was thinking……..please carry on writing…………
thankx
sure @ Naveen, Thanks for Your Feedback.
Thanks man, this is really informative. Keep up the good work.
Thanks @ aaron, for your feedback, which means a lot for us.
hi,im fresher.about to learn LINUX system administration.could you please provide books for me
Check our Free ebook section at top, you will find plenty of good ebooks on Linux Administration.
Thanks. It’s very clear.
:) @ javier, Thanks for your feedback.
Nice post. I am new-bee in linux and found of shell script. Your blog empowering my learning. Thanks for sharing your knowledge. Looking forward more quality post….
Thanks @ Tapan Kumer Das, for your valueable feedback. we will continue to produce such articles.
Thank You Avishek Kumar
:) Thanks Srinivas for your thanks.
Hi Avishek,
I was going good with the first 2 script but the 3rd gives me headache.
When I am executing the script, it gives me eror message:
./name.sh: line 8: s: command not found
Hey whats time to say goodbey
Do you know what causes the fault?
“I have a MacbookPro”
@ kisszso, the script is perfectly ok. You need to copy and paste the above code, and nothing else, save it as anything.sh and run it as ./script_name.sh
well “time to say goodbey” is a echo statement and will be printed on standard output as it is
Hi ,
Nice article ,But is this limit to be a perfect script writer …..Or more please write
Sure @ Lalit, we have already published a series of articles and will continue to produce such high quality articles, in future.
Wowww!!!
Yet another very nice and informative article published on this website..
A big THANKS from my side..
Thanks @ Mandar for such a valueable feedback
Hi, keep them articles coming!
Question: is the semicolon optional at the end of each line? Is there a rule when you have to put a ; or when you can omit it?
; (semicolon) after a command forces the next command to wait till the previous command has finished.
The best to learn is to experiment. Why don’t u remove the semicolon and run the script, this way u’ll learn something you will really proud of
At the end of a line a semicolon does nothing; it can be omitted.
In the middle of a line it separates commands.
Hey there,
great guide.
Is it possible to write some posts for your site?
Would be great.
best regards,
daniel
Yes! you can write articles and Submit Here.
Great Article, Thank You :)
Nice, simple graphic to go with the article. Thanks.
nice article…keep writing for us..thanks
Thanks satyamdhaker, comment of this kind encourages us.
i really appreciate you work please keep writing articles for us site bookmarked* :)