In this article, we will take a look at the Python Sys Module. There are variables and functions that are maintained by the interpreter and the sys module provides a way of interacting with them. These variables are available until the interpreter is alive. We will have a glance at some of the commonly used sys functions.
To work with the sys module you have to first import the module.
sys.version – This stores the information about the current version of python.
$ python3 >>> import sys >>> sys.version

sys.path – Path variable stores the directory path in the form of a list of strings. Whenever you import a module or run a program using a relative path, python interpreter search for the necessary module or script using the path variable.
Path index stores the directory containing the script that was used to invoke the Python interpreter at the index “Zero”. If the interpreter is invoked interactively or if the script is read from standard input, path[0] will be an empty string.
>>> sys.path

When invoking the script the path[0] stores the directory path.
$ vim 1.py $ python3 1.py

If you have modules in a custom directory then you can add the directory path to the path variable using a path.append() method (since the path is a list object we are using the list method “append”).
$ python3 >>> import sys >>> sys.path >>> sys.path.append('/root/test/') >>> sys.path
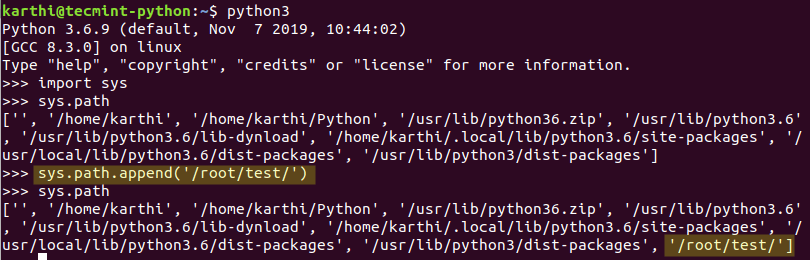
sys.argv – argv is used to pass run time arguments to your python program. Argv is a list that stores the script name as the 1st value followed by the arguments we pass. Argv values are stored as type string and you have to explicitly convert it according to your needs.
>>> sys.argv
When you run below snippet, the end value of range function is passed via sys.argv[1] as 10 and few other values are also passed to print the list of argv values at the end of the program.
#!/usr/bin/python3 import sys for x in range(1,int(sys.argv[1])): print(x) # Print all the arguments passed print("Arguments passed:",sys.argv)
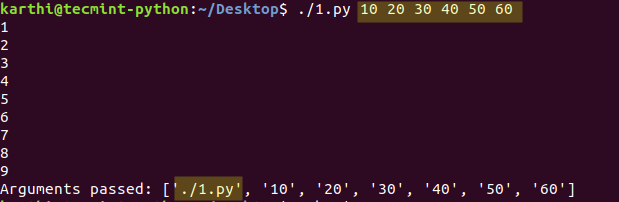
sys.executable – Prints the absolute path of the python interpreter binary.
>>> sys.executable '/usr/bin/python3'
sys.platform – Prints the os platform type. This function will be very useful when you run your program as a platform dependent.
>>> sys.platform 'linux'
sys.exit – Exit the interpreter by raising SystemExit(status). By default, status is said to be Zero and is said to be successful. We can either use an integer value as Exit Status or other kinds of objects like string(“failed”) as shown in the below example.
Below the sample, a snippet is used to check if the platform is windows and then run the code. If not raise exit() function.
#!/usr/bin/python3 import sys if sys.platform == 'windows': # CHECK ENVIRONMENT #code goes here pass else: print("This script is intended to run only on Windows, Detected platform: ", sys.platform) sys.exit("Failed")

sys.maxsize – This is an integer value representing maximum value a variable can hold.
On a 32-bit platform it is 2**31 - 1 On a 64-bit platform it is 2**63 - 1
Wrapup
We have seen some of the important functions of the sys module and there are a lot more functions. Until we come up with the next article you can read more about the sys module here.