When you write a Bash script, sometimes you want to add comments to explain what certain parts of the script do.
In Bash scripting, there are several ways to add comments, and in this article, we’ll explore different methods to do just that.
1. Single Line Comment in Bash
The most common way to add comments in Bash scripts is by using the #
symbol. Anything that comes after the #
on a line is considered a comment and is ignored by the Bash interpreter.
Here’s an example:
#!/bin/bash # This is a comment echo "Hello, World!"
In this script, ‘# This is a comment‘ is a comment, and it doesn’t affect the execution of the script.
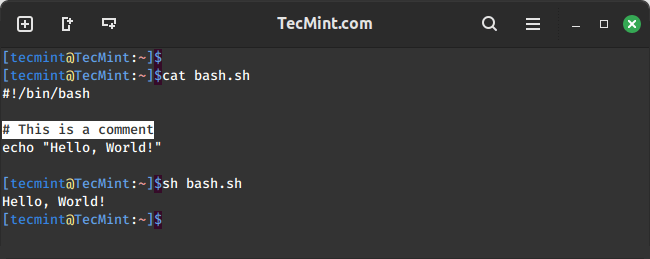
2. Inline Comments in Bash
You can also add comments on the same line as a command using the #
symbol, as these comments appear alongside the command or code statement.
Here’s an example:
#!/bin/bash echo "Hello, World!" # This is an inline comment
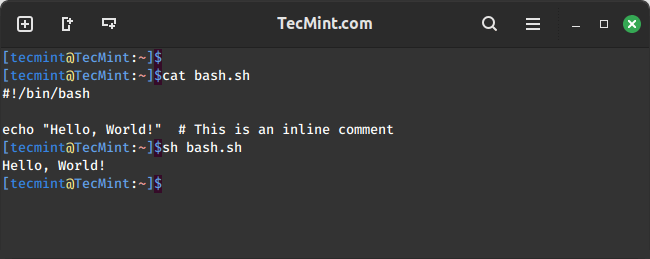
3. Multi-line Comments in Bash
In Bash scripting, the language doesn’t have a built-in multi-line comment syntax like some other programming languages.
However, you can simulate multi-line comments by placing a #
at the beginning of each line you want to comment out.
Here’s an example:
#!/bin/bash # This is a multi-line comment. # It spans across several lines. # These lines will not be executed. echo "Hello, World!"
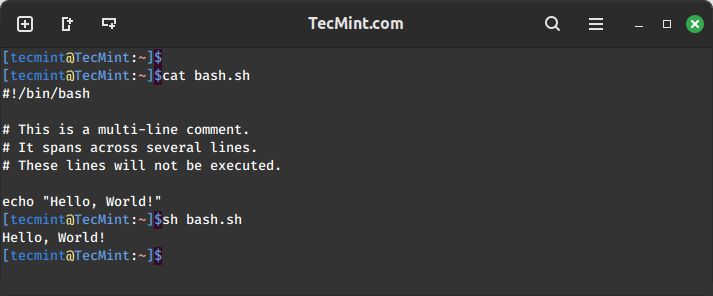
4. Multi-Line Comments with “: <<” Operator
You can also create multi-line comments using the : <<
operator, which allows you to add comments spanning multiple lines.
Here’s an example:
#!/bin/bash : <<'COMMENT' This is a multi-line comment. It can span several lines. This text will not be executed. COMMENT echo "Hello, World!"
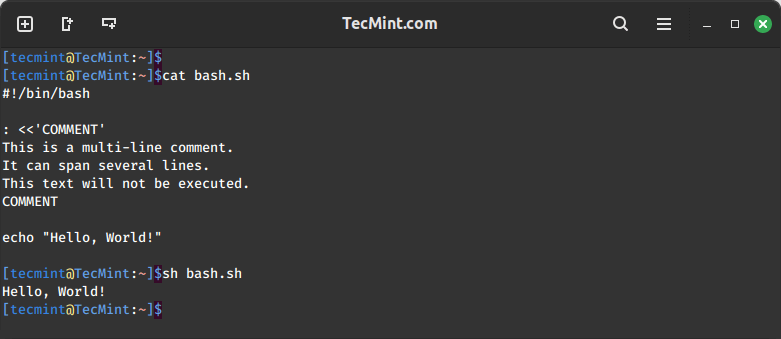
5. Add Comments in Bash Using Heredocs
Heredocs are another way to add comments in Bash scripts, as they are similar to the : <<
method but offer more flexibility.
Here’s an example:
#!/bin/bash <<COMMENT This is a heredoc comment. It can also span multiple lines. This text will not be executed. COMMENT echo "Hello, World!"
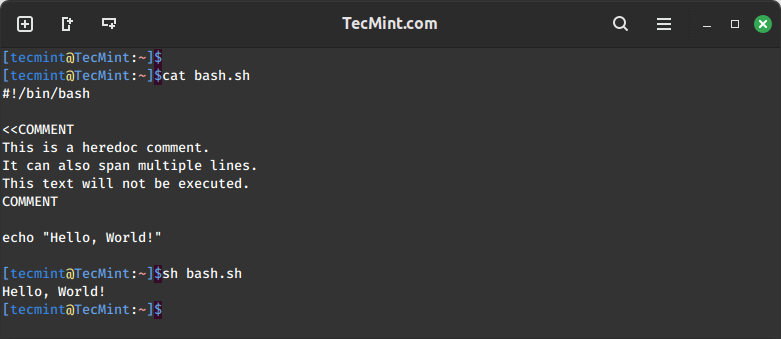
6. Add Comments in Bash Using : as a No-Op Command
In Bash, the ":"
symbol is a no-op command that does nothing. You can use it to add comments in your script.
Here’s an example:
: This is a comment using the no-op command echo "Hello, World!"
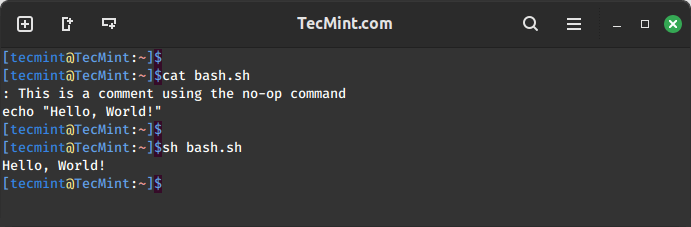
Conclusion
Adding comments to your Bash scripts is essential for improving readability and understanding. Whether you use the #
symbol for single-line comments, the : <<
operator for multi-line comments, heredocs, or inline comments, it’s crucial to document your code effectively.
By choosing the right method for your comments, you can make your Bash scripts more understandable for yourself and others who might read your code.