In this article, we will take a look at how to use a break and continue in bash scripts. In bash, we have three main loop constructs (for, while, until). Break and continue statements are bash builtin and used to alter the flow of your loops. This concept of break and continue are available in popular programming languages like Python.
$ type -a break continue

Exit the loop with a Break Statement
The break statement will exit out of the loop and control is passed to the next statement in the loop. You can run the help command to get some information about the break statement.
$ help break
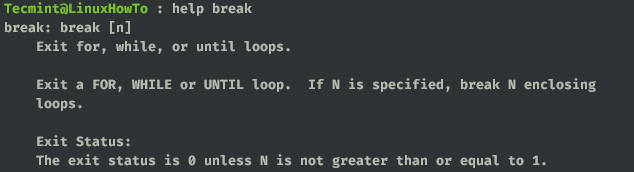
The basic syntax of break.
$ break [n] n is optional
Take a look at the below example. This is a simple for loop that iterates over a range of values from 1 to 20 in an incremental step of 2. The conditional statement will evaluate the expression and when it is true($val = 9) then it will run the break statement and the loop will be terminated skipping the remaining iterations.
#!/usr/bin/bash for val in {1..20..2} do If [[ $val -eq 9 ]] then break else echo "printing ${val}" fi done
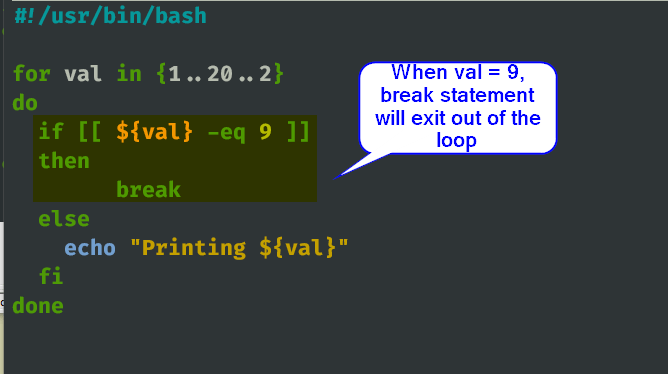
Skip an Iteration with continue Statement
What if you don’t want to completely exit out of the loop but skip the block of code when a certain condition is met? This can be done with a continue statement. The continue statement will skip the execution of the code block when a certain condition is met and the control is passed back to the loop statement for the next iteration.
To access help.
$ help continue
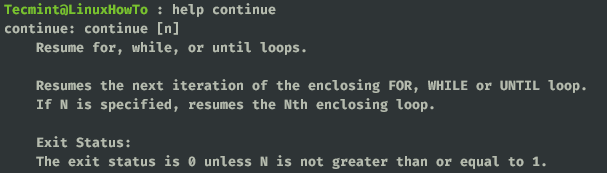
Take a look at the below example. This is the same example we used to demonstrate the break statement. Now when Val is evaluated to nine then the continue statement will skip all the remaining blocks of code and pass the control to for loop for the next iteration.
#!/usr/bin/bash for val in {1..20..2} do If [[ $val -eq 9 ]] then continue fi echo "printing ${val}" done
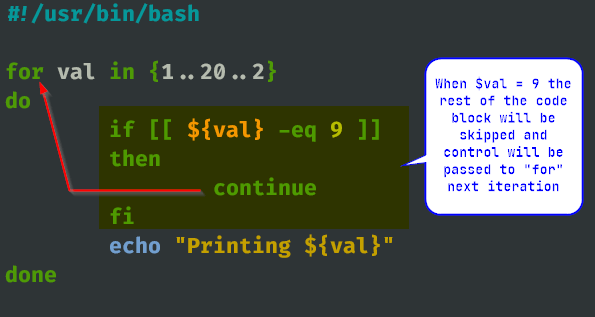
If you knew python then break and continue behavior is the same in python too. But python provides one more loop control statement called a pass.
Pass is like a null statement and the interpreter will read it but will not perform any operation. It simply results in no operation. Bash doesn’t provide a similar statement but we can emulate this behavior using true keyword or colon(:). Both true and colon are shell builtin and not perform any operation.
$ type -a : true

Take a look at the below example. When a conditional statement is evaluated to be true($val = 9) then the true statement will do nothing and the loop will continue.
#!/usr/bin/bash for val in {1..20..2} do If [[ $val -eq 9 ]] then true fi echo "printing ${val}" done
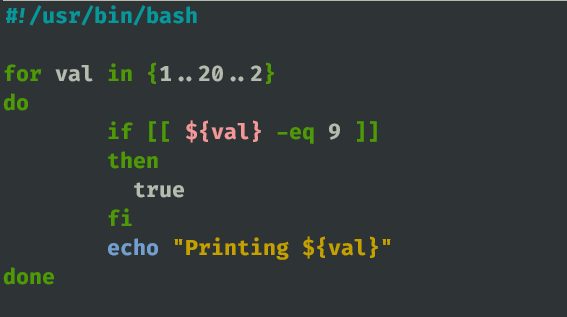
That’s it for this article. We would love to hear your valuable feedback and any tips you have.
Thanks for that good explanation.
Cheers!