In bash for, while, and until are three loop constructs. While each loop differs syntactically and functionally their purpose is to iterate over a block of code when a certain expression is evaluated.
Until loop is used to execute a block of code until the expression is evaluated to be false. This is exactly the opposite of a while loop. While loop runs the code block while the expression is true and until loop does the opposite.
until [ expression ] do code block ... ... done
Let’s break down the syntax.
- To start the loop you should use until keyword followed by an expression within single or double braces.
- The expression should be evaluated as false until to start running the code block.
- The actual block of code is placed between do and done.
In this short article, you will learn how to use until loop in your shell scripts using the following examples.
Create an Infinite Loop in Scripts
You can create an infinite loop using a false statement as an expression. When you try to simulate infinite loops try to use sleep which will pass the script periodically.
count=0 until false do echo "Counter = $count" ((count++)) sleep 2 done
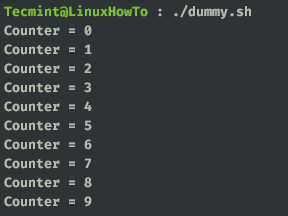
Create Single Line Statements
You can create single-line loop statements. Take a look at the below code. This is the same as our first infinite loop example but in a single line. Here you have to use a semicolon (;)
to terminate each statement.
# until false; do echo "Counter = $count"; ((count++)); sleep 2; done

Alter Flow with break and continue Statement
You can use a break and continue statements inside while loop. The break statement will exit out of the loop and will pass the control to the next statement while the continue statement will skip the current iteration and start the next iteration in the loop.
I am using the same infinite loop example. Here when the count is equal to five continue statement will jump over to the next iteration skipping the rest of the loop body. Similarly, the loop breaks when the count is equal to or greater than 10.
count=0 until false do ((count++)) if [[ $count -eq 5 ]] then continue elif [[ $count -ge 10 ]] then break fi echo "Counter = $count" done
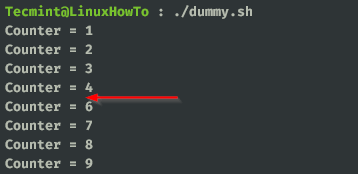
That’s it for this article. We will catch you with another interesting article soon ‘until‘ then keep reading and keep supporting us.