In programming languages, Loops are essential components and are used when you want to repeat code over and over again until a specified condition is met.
In Bash scripting, loops play much the same role and are used to automate repetitive tasks just like in programming languages.
In Bash scripting, there are 3 types of loops: for loop, while loop, and until loop. The three are used to iterate over a list of values and perform a given set of commands.
In this guide, we will focus on the Bash For Loop in Linux.
Table of Contents
Bash For Loop Syntax
As mentioned earlier, the for loop iterates over a range of values and executes a set of Linux commands.
For loop takes the following syntax:
for variable_name in value1 value2 value3 .. n do command1 command2 commandn done
Let us now check a few example usages of the bash for loop.
Bash For Loop Example
In its simplest form, the for loop takes the following basic format. In this example, the variable n
iterates over a group of numerical values enclosed in curly braces and prints out their values to stdout.
for n in {1 2 3 4 5 6 7}; do echo $n done
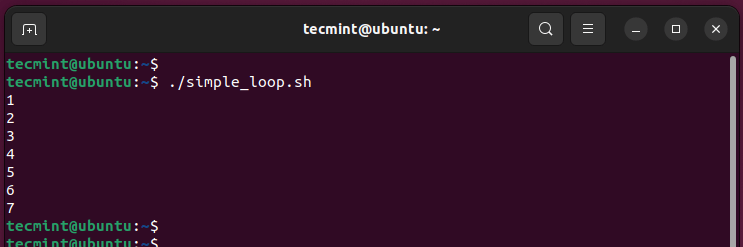
Bash For Loop with Ranges
In the previous examples, we explicitly listed the values to be iterated by the for loop, which works just fine. However, you can only imagine how cumbersome and time-consuming a task it would be if you were to iterate over, for example, a hundred values. This would compel you to type all the values from 1 to 100.
To address this issue, specify a range. To do so, specify the number to start and stop separated by two periods.
In this example, 1 is the first value whilst 7 is the last value in the range.
#!/bin/bash for n in {1..7}; do echo $n done
Once the shell script is executed, all the values in the range are listed, similar to what we had in simple loops.
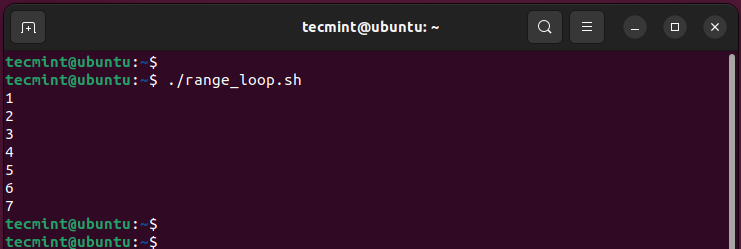
Additionally, we can include a value at the end of the range that is going to cause the for loop to iterate through the values in incremental steps.
The following bash script prints the values between 1 and 7 with 2 incremental steps between the values starting from the first value.
#!/bin/bash for n in {1..7..2}; do echo $n done
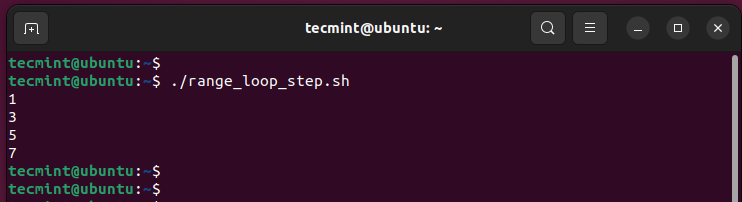
From the above example, you can see that the loop incremented the values inside the curly braces by 2 values.
Bash For Loops with Arrays
You can also easily iterate through values defined in an array using a for Loop. In the following example, the for loop iterates through all the values inside the fruits array and prints them to stdout.
#!/bin/bash fruits=("blueberry" "peach" "mango" "pineapple" "papaya") for n in ${fruits[@]}; do echo $n done
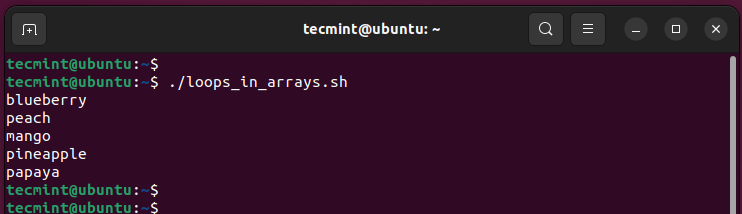
The @
operator accesses or targets all the elements. This makes it possible to iterate over all the elements one by one.
In addition, you can access a single element by specifying its position within the array.
For example to access the “mango” element, replace the @
operator with the position of the element in the array (the first element starts at 0, so in this case, “mango” will be denoted by 2).
This is what the for loop looks like.
#!/bin/bash fruits=("blueberry" "peach" "mango" "pineapple" "papaya") for n in ${fruits[2]}; do echo $n done
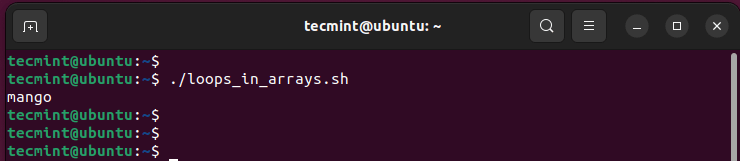
Bash C Style For Loop
You can use variables inside loops to iterate over a range of elements. This is where C-styled for loops come in. The following example illustrates a C-style for loop that prints out a list of numerical values from 1 to 7.
#!/bin/bash n=7 for (( n=1 ; n<=$n ; n++ )); do echo $n done
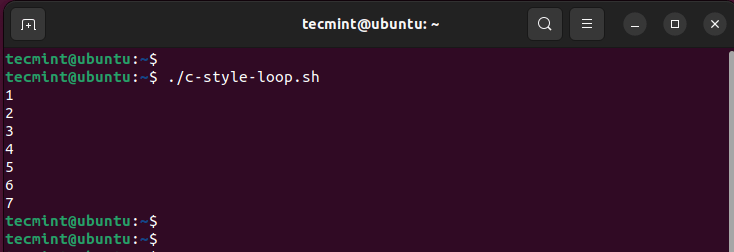
Bash C-styled For Loops With Conditional Statements
You can include conditional statements inside C-styled for loops. In the following example, we have included an if-else statement that checks and prints out even and odd numbers between 1 and 7.
#!/bin/bash for (( n=1; n<=7; n++ )) do # Check if the number is even or not if (( $n%2==0 )) then echo "$n is even" else echo "$n is odd" fi done
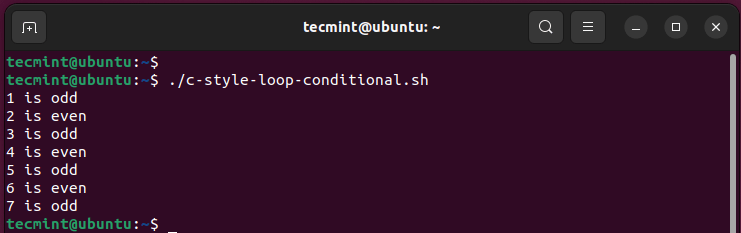
Use the ‘Continue’ statement with Bash For Loop
The ‘continue‘ statement is a built-in command that controls how a script runs. Apart from bash scripting, it is also used in programming languages such as Python and Java.
The continue statement halts the current iteration inside a loop when a specific condition is met, and then resumes the iteration.
Consider the for loop shown below.
#!/bin/bash for n in {1..10} do if [[ $n -eq '6' ]] then echo "Target $n has been reached" continue fi echo $n done
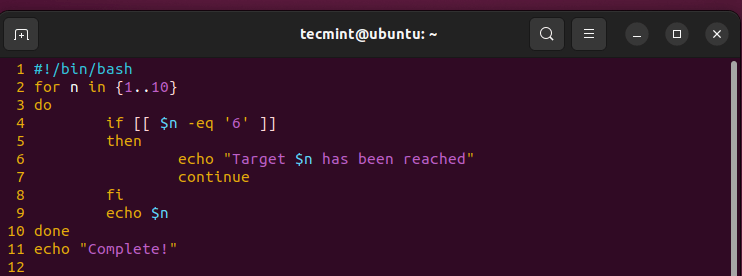
This is what the code does:
- Line 2: Marks the beginning of the for loop and iterate the variable n from 1 to 10.
- Line 4: Checks the value of n and if the variable is equal to 6, the script echoes a message to stdout and restarts the loop at the next iteration in line 2.
- Line 9: Prints the values to the screen only if the condition in line 4 is false.
The following is the expected output after running the script.
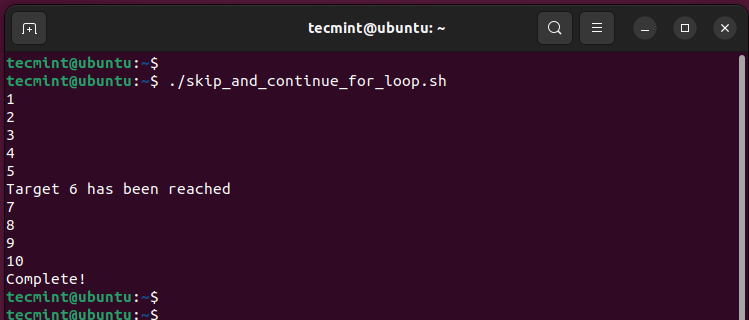
Use the ‘break’ statement with Bash For Loop
The ‘break’ statement, as the name suggests, halts or ends the iteration when a condition is met.
Consider the For loop below.
#!/bin/bash for n in {1..10} do if [[ $n -eq '6' ]] then echo "Target $n has been reached" break fi echo $n done echo "All done"
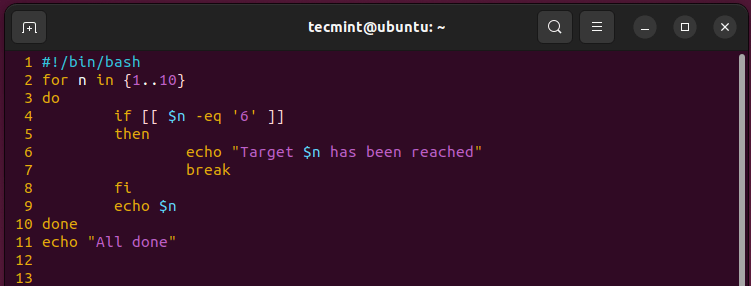
This is what the code does:
- Line 2: Marks the beginning of the for loop and iterate the variable n from 1 to 10.
- Line 4: Checks the value of n and if the variable is equal to 6, the script echoes a message to stdout and halts the iteration.
- Line 9: Prints the numbers to the screen only if the condition in line 4 is false.
From the output, you can see that the loop stops once the variable meets the condition of the loop.
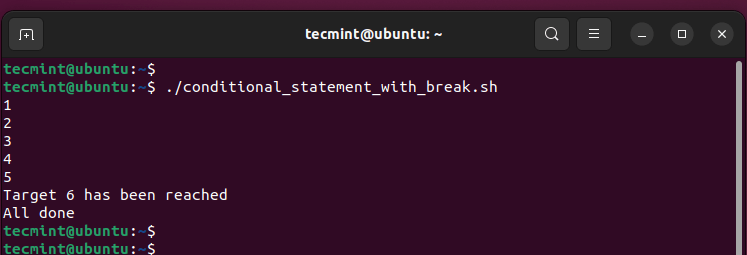
Conclusion
That was a tutorial about Bash For loops. We hope you found this insightful. Feel free to weigh in with your feedback.
Please test your examples. The very first example you give does not do what you say it does. I print the curly brackets, which is not what you show.
I’m going back to c++. At least that does what it says on the tin!