In the ever-changing world of software development, efficient remote server management is crucial. Whether you are a system administrator, a software engineer, or a software developer, being able to run Linux commands on remote servers is a common task.
This is where Fabric, a high-level Python library that is designed to execute Linux commands remotely over SSH, makes it an indispensable tool for anyone who needs to manage remote systems while leveraging the power of Python.
What is Fabric?
Fabric is a versatile Python library that simplifies the process of running shell commands on remote servers over SSH connections. It was initially developed by Jeff Forcier and later became an open-source project.
It provides a high-level, Pythonic interface for working with remote systems, allowing you to automate tasks, deploy code, and manage configurations across multiple servers effortlessly.
Why Use Fabric?
There are several compelling reasons to choose Fabric for your remote command execution needs:
- Pythonic Approach – Fabric adheres to Python’s philosophy, making it easy for developers and system administrators to write scripts in a language they are already familiar with.
- SSH and Paramiko – Fabric relies on the Paramiko library to handle SSH connections, which provides a secure and reliable way to execute commands remotely.
- Task-Based Workflow – Fabric encourages a task-oriented approach to remote command execution. You define tasks, which are essentially Python functions, and Fabric takes care of executing them on remote servers.
- Use Cases – Fabric is a versatile tool suitable for a wide range of use cases, including deploying applications, managing server configurations, and running system maintenance tasks.
In this guide, we will cover the steps to introduce and get started with using Fabric to enhance server administration for groups of servers.
How to Install Fabric Automation Tool in Linux
An important characteristic of fabric is that the remote machines which you need to administer only need to have the standard OpenSSH server installed.
You only need certain requirements installed on the server from which you are administering the remote servers before you can get started.
Requirements:
- Python 2.5+ with the development headers
- Python-setuptools and pip (optional, but preferred) gcc
Fabric is easily installed using the pip package manager, but you may also prefer to choose your default package manager yum, dnf, or apt/apt-get to install the fabric package, typically called fabric or python-fabric.
Install Fabric in RHEL Systems
On RHEL-based distributions such as CentOS Stream, Rocky Linux, and AlmaLinux, you must have the EPEL repository installed and enabled on the system to install the fabric package.
sudo dnf install epel-release sudo dnf install fabric
Install Fabric in Debian Systems
On Debian-based distributions such as Ubuntu and Linux Mint users can simply do apt to install the fabric package as shown:
sudo apt install fabric
Install Fabric Using PiP
If fabric is not available from your system’s repositories, you can use pip to install it as shown.
sudo yum install python3-pip [On RedHat based systems] sudo dnf install python3-pip [On Fedora 22+ versions] sudo apt install python3-pip [On Debian based systems]
Once pip has been installed successfully, you may use pip to grab the latest version of fabric as shown:
pip3 install fabric
How to Use Fabric to Automate Linux Administration Tasks
Let’s get started on how to use Fabric. As part of the installation process, a Python script called 'fab'
has been added to a directory in your system’s PATH. The 'fab'
script handles all the tasks when using Fabric.
Run Linux Commands Locally
By convention, you need to start by creating a Python file called fabfile.py
using your favorite text editor. Remember you can give this file a different name as you wish but you will need to specify the file path as follows:
fab --fabfile /path/to/the/file.py
Fabric uses 'fabfile.py'
to run tasks, the 'fabfile'
should be located in the same directory where you execute the Fabric tool.
Example 1: Let’s create a basic Hello World
first.
# vi fabfile.py
Add these lines of code to the file.
def hello(): print('Hello world, Tecmint community')
Save the file and run the command below.
# fab hello
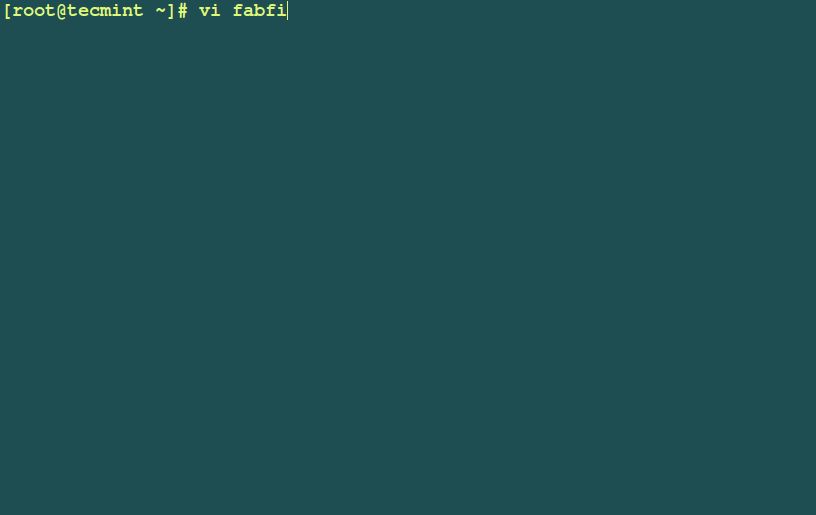
Let us now look at an example of a fabfile.py to execute the uptime command on the local machine.
Example 2: Open a new fabfile.py file as follows:
# vi fabfile.py
And paste the following lines of code in the file.
#! /usr/bin/env python from fabric.api import local def uptime(): local('uptime')
Then save the file and run the following command:
# fab uptime
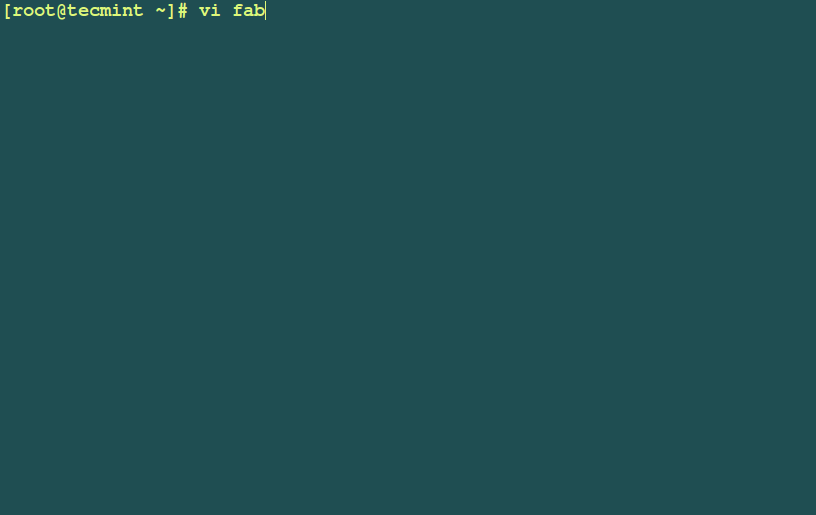
Run Linux Commands Remotely Over SSH
The Fabric API uses a configuration dictionary which is Python’s equivalent of an associative array known as env
, which stores values that control what Fabric does.
The env.hosts
is a list of servers on which you want to run Fabric tasks. If your network is 192.168.0.0 and wish to manage hosts 192.168.0.2 and 192.168.0.6 with your fabfile, you could configure the env.hosts as follows:
#!/usr/bin/env python from fabric.api import env env.hosts = [ '192.168.0.2', '192.168.0.6' ]
The above line of code only specifies the hosts on which you will run Fabric tasks but do nothing more. Therefore you can define some tasks, Fabric provides a set of functions that you can use to interact with your remote machines.
Although there are many functions, the most commonly used are:
- run – which runs a shell command on a remote machine.
- local – which runs the command on the local machine.
- sudo – which runs a shell command on a remote machine, with root privileges.
- Get – which downloads one or more files from a remote machine.
- Put – which uploads one or more files to a remote machine.
Example 3: To echo a message on multiple machines create a fabfile.py
such as the one below.
#!/usr/bin/env python from fabric.api import env, run env.hosts = ['192.168.0.2','192.168.0.6'] def echo(): run("echo -n 'Hello, you are tuned to Tecmint ' ")
To execute the tasks, run the following command:
# fab echo
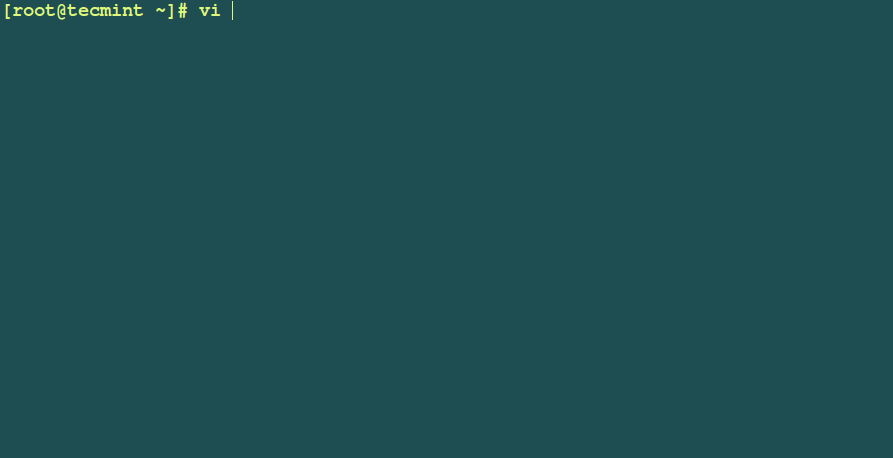
Example 4: You can improve the fabfile.py
which you created earlier on to execute the uptime command on the local machine so that it runs the uptime command and also checks disk usage using the df command on multiple machines as follows:
#!/usr/bin/env python from fabric.api import env, run env.hosts = ['192.168.0.2','192.168.0.6'] def uptime(): run('uptime') def disk_space(): run('df -h')
Save the file and run the following command:
# fab uptime # fab disk_space
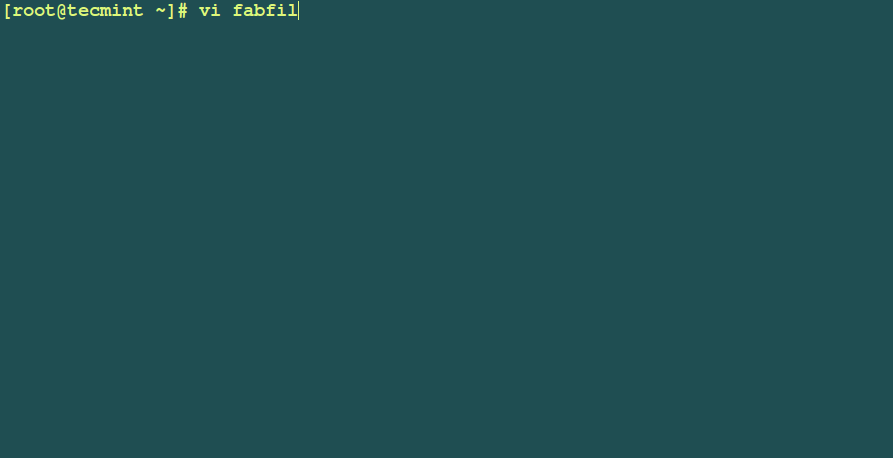
Automatically Install LAMP Stack on Remote Linux Server
Example 4: Let us look at an example of deploying a LAMP (Linux, Apache, MySQL/MariaDB, and PHP) server on a remote Linux server.
We shall write a function that will allow LAMP to be installed remotely using root privileges.
For RHEL/CentOS and Fedora
#!/usr/bin/env python from fabric.api import env, run env.hosts = ['192.168.0.2','192.168.0.6'] def deploy_lamp(): run ("yum install -y httpd mariadb-server php php-mysql")
For Debian/Ubuntu and Linux Mint
#!/usr/bin/env python from fabric.api import env, run env.hosts = ['192.168.0.2','192.168.0.6'] def deploy_lamp(): sudo("apt-get install -q apache2 mysql-server libapache2-mod-php php-mysql")
Save the file and run the following command:
# fab deploy_lamp
Note: Due to the large output, we can’t create a screencast (animated gif) for this example.
Now you can able to automate Linux server management tasks using Fabric and its features and examples given above…
Fabric Useful Options
- You can run
fab --help
to view helpful information and a long list of available command line options. - An important option
--fabfile=PATH
that helps you to specify a different Python module file to import other thanfabfile.py
. - To specify a username to use when connecting to remote hosts, use the
--user=USER
option. - To use a password for authentication and/or sudo, use the
--password=PASSWORD
option. - To print detailed info about command NAME, use
--display=NAME
option. - To view formats use
--list
option, choices: short, normal, nested, use the--list-format=FORMAT
option. - To print a list of possible commands and exit, include the
--list
option. - You can specify the location of the config file to use by using the
--config=PATH
option. - To display a colored error output, use
--colorize-errors
. - To view the program’s version number and exit, use the
--version
option.
Summary
Fabric is a powerful Python library that streamlines remote command execution over SSH, providing a user-friendly Pythonic approach. Its ability to simplify complex tasks and automate system management makes it a valuable tool for system administrators, developers, and DevOps professionals.
Whether you’re managing a single server or orchestrating a large-scale deployment, Fabric can help you get the job done efficiently and effectively.
Interesting tool, I heard a lot from educational sites before but…
suppose 2 virtual machines hosting many client sites, say one in New York (NY) and another in Berlin (B).
Suppose only your NY machine provides visual root login and works as a shepherd. How would you manage your NY or B machine?
Quite easy: 1) create the php login subsystem in both machnes, 2) sftp bash scripts in both machnes, 3) create the interface between php and bash scripts in NY machine, 4) call commands on shepard that will be forwarded to client server. Done!
The shell is powerfull, the only danger comes from login hijacking but then you have https, ip checking, ip black/white listing etc.
Tell you the truth it worths making a bash template script with extended use of the source bash command and there you have it: config files, parameters in files, utility files (is integer, is character), task specific files etc.
Nothing helpful messing with another language on top of bash I think or…. you can mesh with just because you can but see it as another project on top of the previous giant list!
That’s a great tool. Thanks for sharing. Found one error though.
In your last example, the second line should read:
from fabric.api import env, sudo
@Indi
Welcome, i think the line is fine. Try to read through the Fabric documentation.
Very useful explanation for newbies and I am a regular follower of your updated thanks
@Ankammarao
Many thanks for appreciating our work and always following us.
which sreencast do you use for this?
@Thorsten,
Those screenshots are in
gif
format and taken using our custom made shell script..