Linux shell scripting is the art of writing small programs (scripts) to automate tasks in the command-line interface, which involves using commands, variables, loops, and conditionals to create efficient and automated workflows.
This article is an extension of our first article, understanding linux shell scripting. In that piece, we introduced you to scripting. Continuing from there, we aim to maintain the momentum and not disappoint you in this article.
Script 1: Drawing a Special Pattern
The following “Special_Pattern.sh” Bash script prompts the user to input a number between 5 and 9. If the input is within this range, the script proceeds to create a pattern of dots in two stages: an ascending pattern and a descending pattern.
The script utilizes nested loops for this purpose, creating a visually appealing pattern. Finally, a message is displayed, indicating support from Tecmint.com whenever assistance is needed.
#!/bin/bash MAX_NO=0 echo -n "Enter a number between (5 to 9): " read MAX_NO if ! [ $MAX_NO -ge 5 -a $MAX_NO -le 9 ]; then echo "Please enter a number between 5 and 9. Try again." exit 1 fi clear for ((i=1; i<=MAX_NO; i++)); do for ((s=MAX_NO; s>=i; s--)); do echo -n " " done for ((j=1; j<=i; j++)); do echo -n " ." done echo "" done ###### Second stage ###################### for ((i=MAX_NO; i>=1; i--)); do for ((s=i; s<=MAX_NO; s++)); do echo -n " " done for ((j=1; j<=i; j++)); do echo -n " ." done echo "" done echo -e "\n\n\t\t\t Whenever you need help, Tecmint.com is always there"
Most of the above ‘keywords‘ should be familiar to you, and many are self-explanatory. For example, ‘MAX‘ sets the maximum value of the variable, ‘for‘ is a loop, and anything within the loop executes repeatedly until the loop is valid for the given input value.
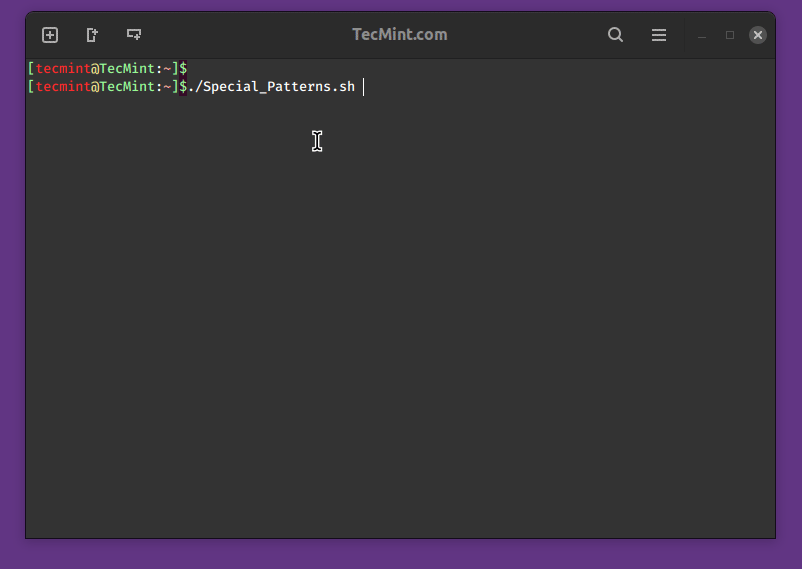
If you are a little aware of any programming language, learning the above script is not difficult, even if you are new to computation, programming, and Linux it is not going to be much more difficult.
Script 2: Creating Colorful Script
The “Colorful.sh” script is a Bash script created to demonstrate the use of ANSI escape codes for adding color and formatting effects to text output in the terminal.
These escape codes provide a simple way to enhance the visual appeal of terminal-based scripts or programs, which display various text styles, colors, and background colors, allowing users to experiment with different combinations.
#!/bin/bash clear echo -e "\e[1mHello World" # bold effect echo -e "\e[5mBlink" # blink effect echo -e "\e[0mHello World" # back to normal echo -e "\e[31mHello World" # Red color echo -e "\e[32mHello World" # Green color echo -e "\e[33mHello World" # Yellow color echo -e "\e[34mHello World" # Blue color echo -e "\e[35mHello World" # Magenta color echo -e "\e[36mHello World" # Cyan color echo -e "\e[0m" # back to normal echo -e "\e[41mHello World" # Red background echo -e "\e[42mHello World" # Green background echo -e "\e[43mHello World" # Yellow background echo -e "\e[44mHello World" # Blue background echo -e "\e[45mHello World" # Magenta background echo -e "\e[46mHello World" # Cyan background echo -e "\e[0mHello World" # back to normal
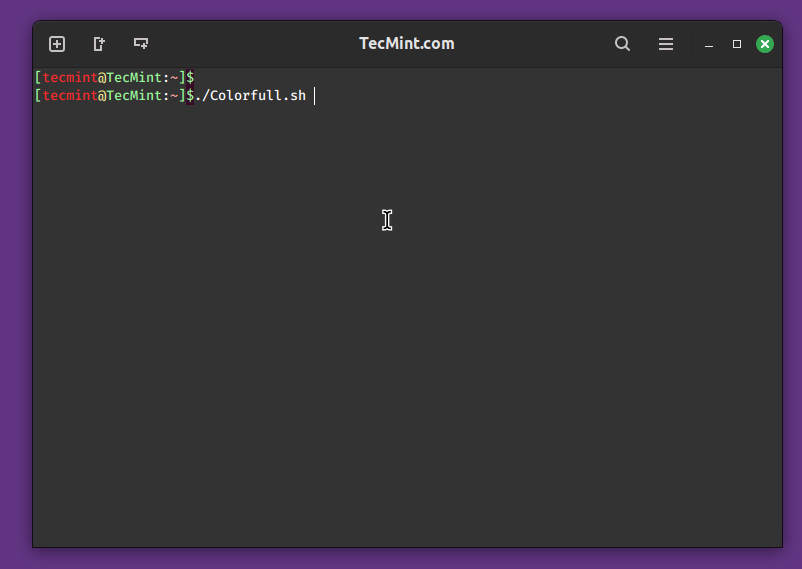
Script 3: Encrypt a File or Directory
The “Encrypt.sh” script is a Bash script designed to provide a simple interface for encrypting a file using the GnuPG (GPG) encryption tool.
The script welcomes the user and prompts them to enter the exact filename, including the extension, of the file or folder they want to encrypt. It then uses GPG to encrypt the specified file, displaying a success message afterward.
Additionally, the script removes the original unencrypted file for added security. Note that the script should be placed in the same directory as the file to be encrypted due to its current limitation.
#!/bin/bash echo "Welcome! I am ready to encrypt a file/folder for you." echo "Currently, I have a limitation. Please place me in the same folder where the file to be encrypted is located." echo "Enter the exact file name with the extension." read file; gpg -c "$file" echo "I have successfully encrypted the file..." # Ask for confirmation before removing the original file read -p "Do you want to remove the original file? (y/n): " confirm if [ "$confirm" == "y" ]; then rm -rf "$file" echo "Original file removed." else echo "Original file was not removed. Exiting without deletion." fi
The command "gpg -c filename"
is used to encrypt a file using GnuPG (GNU Privacy Guard) with symmetric encryption and the command "gpg -d filename.gpg > filename"
is used to decrypt a GPG-encrypted file and save the decrypted content into a new file.
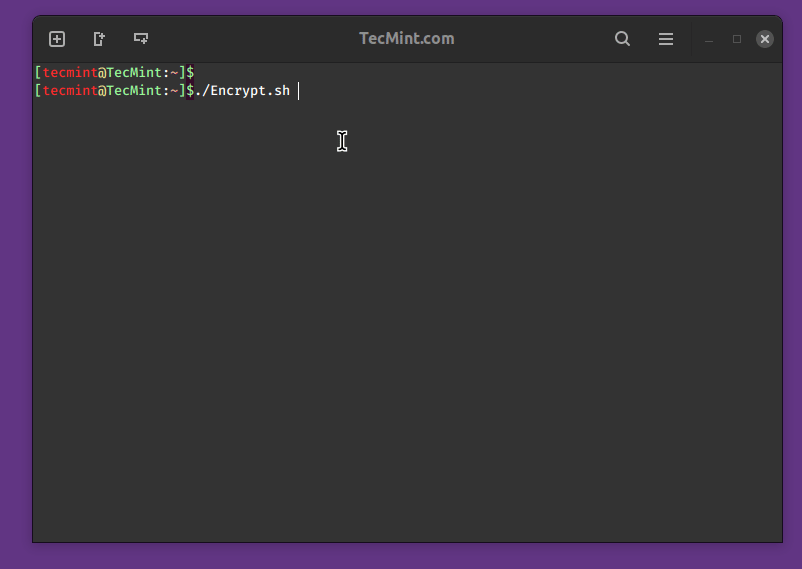
Script 4: Linux Server Monitor Script
The “Server-Health.sh” script is a Bash script designed to provide a comprehensive overview of the health and performance of a server.
When executed, the script gathers various system-related information and presents it in a structured format that includes uptime, currently connected users, disk and memory usage, list open ports, network connections, running processes, and system statistics.
#!/bin/bash date; echo "uptime:" uptime echo "Currently connected:" w echo "--------------------" echo "Last logins:" last -a |head -3 echo "--------------------" echo "Disk and memory usage:" df -h | xargs | awk '{print "Free/total disk: " $11 " / " $9}' free -m | xargs | awk '{print "Free/total memory: " $17 " / " $8 " MB"}' echo "--------------------" start_log=`head -1 /var/log/messages |cut -c 1-12` oom=`grep -ci kill /var/log/messages` echo -n "OOM errors since $start_log :" $oom echo "" echo "--------------------" echo "Utilization and most expensive processes:" top -b |head -3 echo top -b |head -10 |tail -4 echo "--------------------" echo "Open TCP ports:" nmap -p- -T4 127.0.0.1 echo "--------------------" echo "Current connections:" ss -s echo "--------------------" echo "processes:" ps auxf --width=200 echo "--------------------" echo "vmstat:" vmstat 1 5
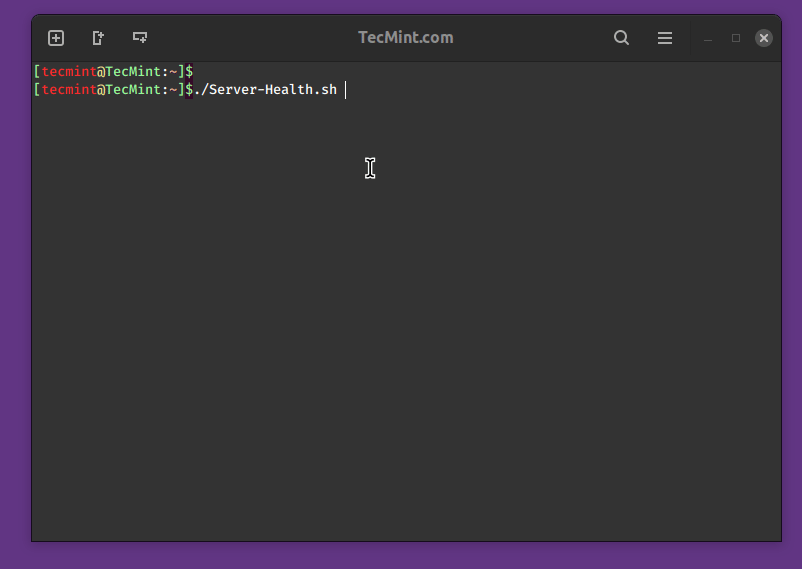
To redirect the output of the “Server-Health.sh” script to a file, you can use the following command:
./Server-Health.sh > server_health_report.txt
Script 5: Linux Disk Space Monitor Script
The “Check-Disk-Space.sh” script is designed to monitor disk space usage on a specified partition of a server. Configurable parameters include the maximum allowed disk space usage percentage (`MAX`), the email address for receiving alerts (`EMAIL`), and the target partition (`PARTITION`).
The script utilizes the df command to gather disk usage information and sends email alerts if the current usage exceeds the defined threshold.
#!/bin/bash # Set the maximum allowed disk space usage percentage MAX=90 # Set the email address to receive alerts [email protected] # Set the partition to monitor (change accordingly, e.g., /dev/sda1) PARTITION=/dev/sda1 # Get the current disk usage percentage and related information USAGE_INFO=$(df -h "$PARTITION" | awk 'NR==2 {print $5, $1, $2, $3, $4}' | tr '\n' ' ') USAGE=$(echo "$USAGE_INFO" | awk '{print int($1)}') # Remove the percentage sign if [ "$USAGE" -gt "$MAX" ]; then # Send an email alert with detailed disk usage information echo -e "Warning: Disk space usage on $PARTITION is $USAGE%.\n\nDisk Usage Information:\n$USAGE_INFO" | \ mail -s "Disk Space Alert on $HOSTNAME" "$EMAIL" fi
Script writing and programming have no limits; you can implement anything as needed. That’s all for now. In my next article, I will introduce you to some different scripting flavors.
I was bothered by one of these scripts (#3 gpg encryption), and strongly suggest at least checking the return code from gpg before removing any file, especially when teaching how to write scripts.
I don’t believe it is good practice to create scripts that remove files without pausing to ask permission first. The rm command has a
-I
option to prompt before deleting files which might be one possibility.A better option might be to decrypt each encrypted file and compare it to the original before removal. I also suggest considering how unexpectedly the script as presented might operate when given file/directory names containing spaces.
I appreciate what you all do here, and understand the intention was to keep it simple. It’s great to teach scripting, but even better to teach defensive scripting. Feel free to take it or leave it, but that’s my two cents.
@Pete,
I have modified a script and included a confirmation prompt, where the script will only proceed with deleting the original file if the user enters
'y'
for yes. If'n'
or any other input is provided, the script will exit without removing the file.This helps prevent accidental deletions and provides a safer user experience.
This is my script. I am a beginner so I just used the old template. It was successful.
Please give the decryption code i had tried writing but not working..
Hi, what does
cut -d % -f1
represent?Hi! Thanks for this article. It is very useful. However, I have found that for Centos6 or Centos7, you have to add
"\"
into the command for the Colourful Script to activate the-e
command.### Below is the decryption script####
Hello,
Actually I tried file encrypt script but when I execute the code it gives me error. Can you please send me how to do it will really appreciate your help.
Thank you
@Qazi,
What error you getting while executing the script code? could you share with me here, so that we can help you out..
gpg -d $file.gpg > decpt.txt
try this it will work.Hi.
You can try this.
Hi, good article. Perhaps when you are searching for OOM messages it is better to search the string “Out of memory” as with “kill” you would find more output then required.
Thanks
Hello,
A friend show me a website named UsiScript (http://www.usiscript.com). It’s a tool that help you to create a bash script, even you don’t know the syntax. It’s simple and fast.
You all guys are the best….
One request to all of you pls explain every field in script for more understanding to the readers.
Thank you all.
can you help me .
how to use counter variable in shell script ?
Pls try and explain each script in details and can u give me the code to decrypt an encrypted script. Thanks guys
Can you hep me to write a bash script that change the passphrase in a *.gpg file which i created before. The situation is that I create a decrypted file with passphrase by follow you above. Now i want to change the passphrase of that decrypted file, is that possible?
Hello
I have tried the script1, I got error “syntax error: unexpected end of file”. I search google, but still not be able fix it.
Can you please advise?
I think for defining colors, it would be much easier if you do:
Afte that:
“text” will be covered in red
Hi Avishek,
Drawing a Special Pattern: Throwing an error
./pattern.sh: 19: ./pattern.sh: Syntax error: Bad for loop variable
I have given 777 permission of the file.
You’re great man, thanks! short and precise!
HI Avishek,
Nice blog , can you please guide me how to create one scripts it will check first memory utilazation if it is high then run command sh -c “sync; echo 1 > /proc/sys/vm/drop_caches”
Hi,
I am new to shell scripting, i hope below script helps
@Paresh,
Thanks for the script, but you should tell us what this script all about, so that other users can aware of it..
@Ravi: my bad :)
We need free ram available on server, for that we can use
free -m
command:Now we will try to print only free ram cloumn:
Using xargs, we can get this data in single line and print only 2nd field:
Now we will store this value in free variable:
You can define a variable “limit“, which will be the minimum amount of ram you want free in system.
Then check if free variable is greater than or equal to limit, if yes then everything is okay else clear cache:
@Paresh,
Good nice script for checking free memory usage on Linux, but you’ve tested this script on all Linux distributions? If it works perfectly on all Linux OS, why not create a small 300 words article on this and publish at Tecmint?
If you planning to write a article on this, here are the guidelines to follow:
Introduce yourself, about script and explain each parameter used in this script and place the script in the article and show real time testing of scripts with screenshot and at the end add conclusion about script..
thanks a mile :)
How to print a time stamp of multiple files from different path?
How do you get time stamp of one file? simply by executing of the below commands.
So how will you print time stamp of more than one file from different location.
Hope it helps!
Hi,
I need a shell script that greps a pattern and return the count of each patterns.
Contents of file:
This is an Apple. Apple is good for health
lemon is a fruit
lemon is in yellow color
This is a banana
Just grep words: Apple, lemon, banana and return no of occurrences.preferably shell script.
Here is my Decription script:
So, by an eof error I’ve lost contens of the encrypted file, ‘pattern.sh.gpg’, and now I’ve got only ‘pattern.sh’ with 0 byte. What did I miss?
@ cagcak
Check point number 1. GnuPG from the link : https://www.tecmint.com/linux-password-protect-files-with-encryption/
This is another article from me where i have written encryption and decryption tool for Linux and here you will get your answer.
If not ping me, i will be here only.
Hello,
this is how i wrote the decrypt script don’t know if thats the correct way though but it worked , the thing is that i couldn’t make the script to make the file to appear in its former name (the filename was hello.txt) so i give the option to make a newfilename.txt.
Any help or advice is welcomed.
Thanks for the tutorials it really helps.
decrypt.sh script follows bellow
#!/bin/bash
echo “Welcome, I am ready to decrypt a file/folder for you”
echo “currently I have a limitation, Place me to the same folder, where the file to be
decrypted is present”
echo “Enter the Exact File Name with extension”
read file;
gpg -d $file>hunter.txt
echo “I have decrypted the file succesfully…”
echo “Now I will be removing the encrypted file”
rm -rf $file
Check point number 1. GnuPG from the link : https://www.tecmint.com/linux-password-protect-files-with-encryption/
This is another article from me where i have written encryption and decryption tool for Linux and here you will get your answer.
If not ping me, i will be here only.
How can I show in Linux when -d parameter is supplied the three user in the system that uses more disk space in their homes. Sort these three user by the disk usage and for each of them show their name, total space and home directory.
Output should be like this:
Disk usage:
Jane 204816/users/Jane
Joe 102420/home/joe
sync 12584 /sbin
Hi Avishek,
I am using Fedora 20 – Heisenberg. I wrote a script but the nested for loop is not working as intended. for the following variables I want to print:
I keep getting the result:
Can you tell me why the nested loop is not working? I would really appreciate your help.
Hi Avishek,
I am a beginner. Would like to learn about shell scripting. What would you suggest to start of with?
Thanks a lot sir for your Great help
Welcome @Hari om
I need one help regarding creating new users script.
Let us know how we can help you?
Hi Avishek Kumar,
Please help me .
I want run one jar file, that jar file and input.property file Application team provided and run that jar weekly once, Before running need to take mysql DB dump backup.
Thanks,
Ram
I didnt understand ur question.
see if it helps
Run Jar fie : java -jar filename.jar
schedule the execution : you need to cron the job.
thnx for this series … am looking forward to working through it … however have struck an issue with the script
Script 1: Drawing a Special Pattern
there appears to be a syntax error (near the ‘Second Stage comment’) that prevents it from running in entirety … which makes it a bit frustrating for noobs … :)
any chance of having a look at it :)
cheers
Geoff
@Geoff:- It’s just identation errors. Even I faced same problem. Just place that line onto new one with single for loop in one line and the problem will be solved. Cheers :)
~ Dushyant
I need some assistance with creating the Decrypt script, this is what I have so far.
It decrypts the file, but doesn’t ask for password, and adds the .gpg and .txt at the end of the file.
My other questions were how to take the output of a script and put i into a file or folder.
Please e-mail me at [email protected]
Check point number 1. GnuPG from the link : https://www.tecmint.com/linux-password-protect-files-with-encryption/
This is another article from me where i have written encryption and decryption tool for Linux and here you will get your answer.
If not ping me, i will be here only.
thank u very mach Eng. Avishek this is very helpful for me..
Welcome Binary!
Hi Avishek,
I need one help regarding Linuc Shell scripting.
Sample file content:
The last data coulmn having milli seconds. for example and from above sample data.
My requriement:
For above sample file, need to check if any of the request will takes more than 25 seconds need to send email alert to the team. How to write script for this scenario. Can you please help me on this .
Thanks
Gowri Shankar
Hi
i am a freser and lookin for job as a administratotlr
i a job?? m mcitp…so will scripting be benificial to get
Dear saiba aka Vaibhav Choudhary.
Shell scripting is a very powerful tool and it will surely help you getting a job.
Good Luck.
hello Avishek,
Thanks for this very helpful tips. can you help on decrypting script?
I just changed the gpg -c to gpg -d but it seems not working, I can still see the file as .gpg
Thank you in advance,
Dodie
I am having issues with the decryption script as well. Any help would be appreciated.
what kind of problem?
let us know.
You are doing very good and understandable job. Keep going :)
P.S Do you have an article, how to install O/S with LEMP or LAMP functions?
Thanks in advance.
Dear const,
we already have published article on setting up LEMP and LAMP server and other related articles.
Here these can be found :
http://www.google.com/cse?cx=partner-pub-2601749019656699:2173448976&ie=UTF-8&q=shell+script&sa=Search&ref=#gsc.tab=0&gsc.q=LAMP%20and%20LEMP
Dear Avishek,
Thank you very much… I had seen your articles about LEMP & LAMP and i had already use it, what i meant in my question above was if you have any article about O/S installation via scripting.
i.e (i imagine that it will be very helpful to install O/S via scripting, if you have 10 machines and you need to make it simultaneously) or if you have any article with examples like these ones that you post it but with installation commands.
Thanks again.
Installing Linux via scripting on several machines at a time?
I think you are talking about setting up of PXE Boot server.
Something like that, is this the best way when you have to install new workstations? i’m asking because i really don’t know how to do it. I have in mind to minimize the time when i want to set up new machines, and i thought that it will be the best if i make a script and run it. I.E specific edition CentOS 6.5 or Ubuntu, create new user & pass with home directory, and the most important is to take backup from them i assume every two or three days so not to worry if someone do something.
Thanks again for your help.
Also Avishek i would like to ask which is the best way to create a script with Lamp/Lemp installation? and if you have any similar article about it.
Hi Avishek,
could you please tell me if you are offering online linux scripting classes, and how much doest it costs.
regrds
Dear Lucky!
we are offering location based training classes and currently we are having it in Mumbai.
Free free to contact Tecmint.com about your needs and corresponding cost.
Keep Connected.
Its really helpful for new learner .Share other scripts from where we get some tips and increase our productivity in our organization
Thanks a lot for sharing your knowledge :)
Thanks @ sumant for the appreciation.
Our Shell Scripting Articles can be found at the location :
http://www.google.com/cse?cx=partner-pub-2601749019656699:2173448976&ie=UTF-8&q=shell+script&sa=Search&ref=#gsc.tab=0&gsc.q=shell%20script&gsc.page=1
Keep connected!
Kudos
Very good article Avishek…thanks a lot..
Welcome @ Rajesh,
Thanks for your Nice words.
Well explained !! Good to start the shell script from here..
Good to know @ Vallinayagam,
Keep connected.
Go thorugh our other post.
how to run the shell programs i’m confuging about the path where it is being located the bin while running the program it will giving an error
Dear Mallinath Swamy,
we described above, in very simple words, how to execute a shell script.
well once again,
cd /path/to/folder
chmod 755 script_name.sh
./script_name.sh
Hope it helps.
Very nice.
Thanks @ Moathodi,
For your appreciation.
Nice article… Thanks !! .. Keep posting :-)
Welcome @ suhailcheroor.
we already have published many articles on scripts. You may have a look at
http://www.google.com/cse?cx=partner-pub-2601749019656699:2173448976&ie=UTF-8&q=script&sa=Search&ref=#gsc.tab=0&gsc.q=script&gsc.page=1
Admin Please delete my post……the data i posted and the displaying data is completely different. Apologies
To those who are confusing about the script 1, below is the actual formatted text
Thank you very much Avishek Kumar…that was wonderfull lecture
Great to hear it, @ Naveen
Awasome work dear…….well explained.
Thanks @ Kirti nandan, for the wonderful words.
superb work avishek…really helpful for me!!!!
Thanks @ nipun, for such an admiration.
I am getting error while running pattern sample script
./drawing2.sh: line 15: syntax error near unexpected token `do’
./drawing2.sh: line 15: `do’
don’t copy and past from the site the scripts are missing characters and the formatting is messed up on some of them.
Use the links – Download Special_Pattern.sh
great ! where is the part one?
We already included Part I in this article above, check once again.
part 1: https://www.tecmint.com/understand-linux-shell-and-basic-shell-scripting-language-tips/
Next article of this series will be available soon, keep visiting Tecmint.com
Hello,
the tips u r giving is very helpful for me, thanks a lot
can u plz put debian based command for Ubuntu
its main configuration file location etc….
ThanKs Hari, for the good words about our Article, we will continue to provide you, valueable articles of this kind. However Your question was not clear, hence we are not able to understand your problem.
Debain based command for ubuntu???
Ubuntu is a derivative of Debian, and more or less all the programs will run on ubuntu as smooth as on Debian. Which Kind of Configuration File you Need to edit?
Please Tell us your problem in details and be clear in language. We will be Happy to help you.
Great work. Keept it up…
Thanks @ Ganesh, for the compliment.
very very good support for beginners and professionals
Thanks Aru.